swift 下拉刷新。加载新数据
时间: 2024-09-24 09:06:34 浏览: 71
在Swift中,下拉刷新是一种常见的用户体验设计,用于当用户拉动列表头部时,表示正在加载新的内容。通常在UITableView或UICollectionView中实现。以下是基本步骤:
1. 首先,在需要刷新功能的控制器中,添加一个`UIScrollViewDelegate`协议,因为下拉刷新通常基于滚动视图。
```swift
class YourViewController: UITableViewController, UIScrollViewDelegate {
```
2. 创建一个布尔值变量来跟踪是否正在刷新,例如`isRefreshing`。
3. 在视图加载完毕后设置滚动视图的代理,并初始化下拉刷新控件。对于`UITableView`,可以使用`UIRefreshControl`;对于`UICollectionView`,则可能需要自定义刷新指示器。
```swift
let refreshControl = UIRefreshControl()
refreshControl.addTarget(self, action: #selector(handleRefresh), for: .valueChanged)
tableView.addSubview(refreshControl)
tableView.refreshControl = refreshControl
// 或者如果你使用的是UICollectionView
collectionView.collectionViewLayout.invalidateLayout()
let refreshIndicator = UIView(frame: CGRect(x: 0, y: collectionView.contentOffset.y + collectionView.contentInset.top, width: collectionView.frame.width, height: 44))
refreshIndicator.backgroundColor = .gray
collectionView.addSubview(refreshIndicator)
```
4. 定义`handleRefresh`方法来处理刷新操作。在这里,你可以取消刷新状态、请求新的数据,并更新表格或集合视图。
```swift
@objc func handleRefresh() {
isRefreshing = true // 设置为正在刷新
// 发送网络请求获取新数据
fetchData { data in
if let newData = data {
self.updateContent(with: newData) // 更新数据
DispatchQueue.main.async {
self.isRefreshing = false // 刷新结束并取消刷新状态
tableView.reloadData() // 对TableView重新布局
// 或者对CollectionView
collectionView.reloadData()
}
} else {
// 没有新数据的情况处理
}
}
}
```
5. 当数据加载完成后,你需要通过`updateContent(with:)`方法替换旧的数据并调用刷新视图的结束方法。
记得在完成实际网络请求前,别忘了检查`isDragging`条件,避免在滑动时误触发刷新。
阅读全文
相关推荐
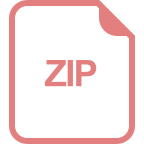
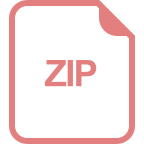
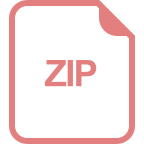

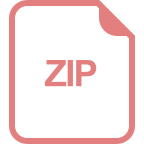
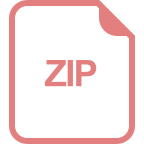
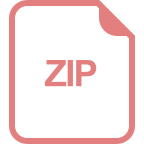
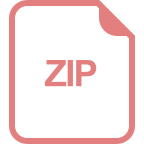
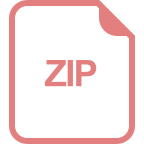
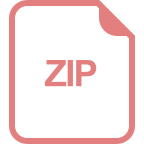
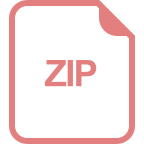
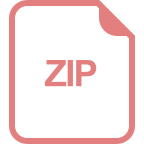
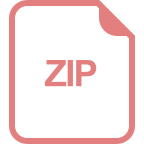
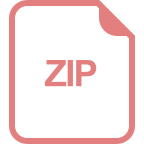
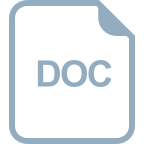
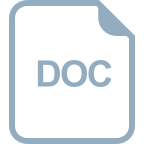
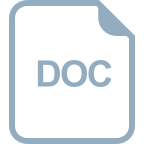
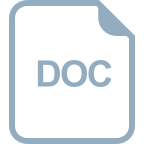