我有一种产品,里面要固定的位置要涂密封胶,我想用opencv, 准备一张没有涂密封胶的图片当做错误样本。之后相机拍照得到的照片与样本图片对比,一致则遗漏涂胶要提示警告。不一致则产品合格。Python语言如何写。
时间: 2024-12-13 08:20:23 浏览: 5
为了实现这个功能,你可以使用OpenCV进行图像处理比较。首先,你需要准备两个步骤:
1. **图像预处理**:
- 从相机获取实时图像和预先定义好的样本图片(无密封胶)。
- 对两幅图片进行预处理,例如灰度化、去噪和大小调整到相同的尺寸。
```python
import cv2
import numpy as np
def preprocess_image(image):
# 将图像转换为灰度
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 应用平滑滤波器去除噪声(如高斯滤波)
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 可选:如果需要调整图片大小,这里缩放成固定尺寸
# resized = cv2.resize(blur, (width, height))
return blur
```
2. **图像对比**:
- 使用像素级别的相似度比较方法,比如SIFT(尺度不变特征变换)、ORB(Oriented FAST and Rotated BRIEF)或直方图比较(如SSIM或计算两幅图像的像素差)。
```python
def compare_images(img1, img2):
# 使用OpenCV的 Structural Similarity Index Measure (SSIM) 或者计算像素差
diff = cv2.compare(img1, img2, cv2.CMP_EQ) # 如果相同返回True,不同返回False
# 或者使用这种方法
# threshold = 0.9 # 相似度阈值,可以根据需求调整
# similarity = cv2.matchTemplate(img1, img2, cv2.TM_CCOEFF_NORMED)
# match_index = np.where(similarity >= threshold)
if diff.all() or similarity.max() < threshold:
return False # 不同
else:
return True # 相同
```
3. **警告逻辑**:
- 当检测到照片中的位置与样本图片不一致时,触发警告提示。
```python
def check_sealant(image_path, sample_image_path):
image = cv2.imread(image_path)
preprocessed_image = preprocess_image(image)
sample_image = preprocess_image(cv2.imread(sample_image_path))
if not compare_images(preprocessed_image, sample_image):
print("警告!产品遗漏涂胶!")
else:
print("产品合格。")
```
然后在主程序中调用`check_sealant`函数:
```python
if __name__ == "__main__":
product_image_path = "path_to_product_image.jpg"
sample_image_path = "path_to_sample_image.jpg"
check_sealant(product_image_path, sample_image_path)
```
阅读全文
相关推荐



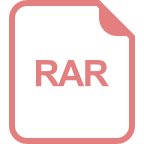



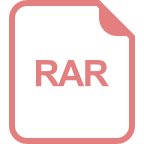


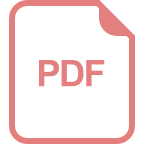



