1. 参考a* 算法核心代码以8 数码问题为例实现a* 算法的求解程序(编程语言不限)
时间: 2023-12-03 08:00:57 浏览: 51
A*算法是一种广泛应用于路径规划和图搜索的算法,也常用于解决八数码问题。该问题是一个三阶矩阵,由1-8和一个空格组成,目标是将初始状态的乱序数码移动到目标状态(12345678)。下面是一个用Python语言实现A*算法解决八数码问题的伪代码:
```python
class Node:
def __init__(self, state, parent, action, cost, heuristic):
self.state = state
self.parent = parent
self.action = action
self.cost = cost
self.heuristic = heuristic
def a_star(initial_state, goal_state):
open_list = [Node(initial_state, None, None, 0, 0)]
closed_list = []
while open_list:
current_node = min(open_list, key=lambda x: x.cost + x.heuristic)
open_list.remove(current_node)
closed_list.append(current_node)
if current_node.state == goal_state:
return current_node
for action in possible_actions(current_node.state):
new_state = apply_action(current_node.state, action)
new_node = Node(new_state, current_node, action, current_node.cost + 1, heuristic(new_state, goal_state))
if new_node in closed_list:
continue
if new_node not in open_list:
open_list.append(new_node)
else:
existing_node = open_list[open_list.index(new_node)]
if new_node.cost < existing_node.cost:
existing_node = new_node
return None
```
上述伪代码简单描述了A*算法的实现过程,其中Node类表示一个状态节点,a_star函数是主要的解决函数。在实际编程中,需要实现可能的动作、应用动作、启发式函数等具体细节。通过实现这些功能,可以完成A*算法对八数码问题的求解程序。
相关推荐
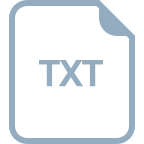
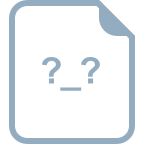














