建立一个链表,包含头节点(头节点不包含数据)用C语言编写
时间: 2024-11-03 10:13:37 浏览: 24
在C语言中,链表是一种动态数据结构,它由一系列节点组成,每个节点包含数据域和指向下一个节点的指针。创建一个简单的单向链表(只包含头节点)的基本步骤如下:
```c
// 定义链表节点结构体
typedef struct Node {
void* data; // 存储数据的地方
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建链表头结点
Node* createHead() {
Node* head = (Node*)malloc(sizeof(Node)); // 分配内存空间
if (head == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
head->next = NULL; // 初始化头节点的下一个指针为空
head->data = NULL; // 头节点一般不存储实际数据
return head;
}
// 添加新节点到链表头部
void insertAtHead(Node** head_ref, void* data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = data;
newNode->next = (*head_ref); // 新节点的下一个指针指向原头节点
*head_ref = newNode; // 更新头节点指针
}
// 打印链表(仅演示,实际应用需要实现)
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%p -> ", current);
current = current->next;
}
printf("NULL\n");
}
// 示例用法
int main() {
Node* head = createHead();
insertAtHead(&head, "Hello"); // 插入字符串
insertAtHead(&head, 42); // 插入整数
printList(head);
return 0;
}
```
阅读全文
相关推荐






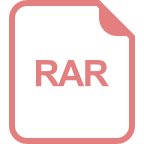











