springboot+mybatisplus多表查询
时间: 2024-02-09 14:06:19 浏览: 38
在Spring Boot中使用MyBatis Plus进行多表查询可以通过以下步骤实现:
1. 首先,确保你已经在你的项目中引入了Spring Boot和MyBatis Plus的依赖。
2. 创建实体类:为每个表创建对应的实体类,并使用注解(如`@Table`、`@Column`等)指定表名和字段名与数据库对应。
3. 创建Mapper接口:为每个实体类创建对应的Mapper接口,继承自MyBatis Plus提供的`BaseMapper`接口。在Mapper接口中,可以使用注解(如`@Select`、`@Update`等)定义SQL语句。
4. 创建Service层:创建对应的Service层接口和实现类,用于处理业务逻辑。在Service层中,可以调用Mapper接口中定义的方法进行数据库操作。
5. 编写多表查询方法:在Service层中,可以编写多表查询的方法。可以使用MyBatis Plus提供的`@TableField`注解指定关联字段,并使用`@TableJoin`注解指定关联表和关联条件。
6. 调用多表查询方法:在Controller层或其他需要的地方,调用Service层中编写的多表查询方法,获取查询结果。
下面是一个简单的示例代码:
```java
// 实体类
@Table(name = "user")
public class User {
@TableId
private Long id;
private String name;
// 其他字段...
}
@Table(name = "order")
public class Order {
@TableId
private Long id;
private Long userId;
private BigDecimal amount;
// 其他字段...
}
// Mapper接口
public interface UserMapper extends BaseMapper<User> {
}
public interface OrderMapper extends BaseMapper<Order> {
}
// Service层
public interface UserService {
List<User> getUsersWithOrders();
}
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Autowired
private OrderMapper orderMapper;
@Override
public List<User> getUsersWithOrders() {
return userMapper.selectList(new QueryWrapper<User>().lambda()
.inSql(User::getId, "SELECT DISTINCT user_id FROM `order`"));
}
}
// Controller层
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public List<User> getUsersWithOrders() {
return userService.getUsersWithOrders();
}
}
```
在上述示例中,我们通过`getUsersWithOrders`方法实现了查询所有用户及其对应的订单信息。在`UserMapper`接口中,我们使用了MyBatis Plus提供的`selectList`方法,并通过`QueryWrapper`和`lambda`表达式实现了多表查询。
相关推荐
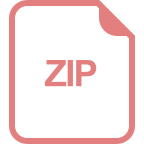
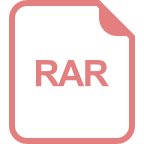
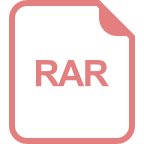














