深度搜索广度搜索c++代码
时间: 2023-10-03 13:02:12 浏览: 182
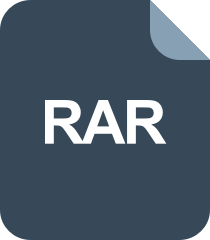
深度搜索c++
深度优先搜索(DFS)是一种从起点开始,沿着一条路径一直搜索到底的算法。它可以使用递归或栈来实现。
以下是C代码示例实现深度优先搜索:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_SIZE 100 // 图的最大节点数
bool visited[MAX_SIZE]; // 记录节点是否被访问过
int adjacencyMatrix[MAX_SIZE][MAX_SIZE]; // 图的邻接矩阵
void dfs(int startNode) {
printf("%d ", startNode); // 打印当前节点
visited[startNode] = true; // 标记当前节点为已访问
for (int i = 0; i < MAX_SIZE; i++) {
if (adjacencyMatrix[startNode][i] && !visited[i]) {
dfs(i); // 递归访问相邻节点
}
}
}
int main() {
// 初始化visited数组和adjacencyMatrix矩阵
int startNode; // 起始节点
printf("请输入起始节点: ");
scanf("%d", &startNode);
printf("深度优先搜索结果:");
dfs(startNode);
return 0;
}
```
广度优先搜索(BFS)是一种从起点开始,按照距离逐层向外搜索的算法。它一般使用队列来实现。
以下是C代码示例实现广度优先搜索:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_SIZE 100 // 图的最大节点数
bool visited[MAX_SIZE]; // 记录节点是否被访问过
int adjacencyMatrix[MAX_SIZE][MAX_SIZE]; // 图的邻接矩阵
void bfs(int startNode) {
int queue[MAX_SIZE];
int front = 0, rear = 0; // 队列的前后指针
printf("%d ", startNode); // 打印起始节点
visited[startNode] = true; // 标记起始节点为已访问
queue[rear++] = startNode; // 将起始节点入队
while (front != rear) {
int currentNode = queue[front++]; // 出队当前节点
for (int i = 0; i < MAX_SIZE; i++) {
if (adjacencyMatrix[currentNode][i] && !visited[i]) {
printf("%d ", i); // 打印当前节点的相邻节点
visited[i] = true; // 标记相邻节点为已访问
queue[rear++] = i; // 将相邻节点入队
}
}
}
}
int main() {
// 初始化visited数组和adjacencyMatrix矩阵
int startNode; // 起始节点
printf("请输入起始节点: ");
scanf("%d", &startNode);
printf("广度优先搜索结果:");
bfs(startNode);
return 0;
}
```
阅读全文
相关推荐
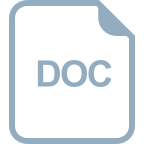
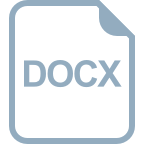
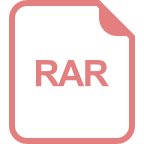
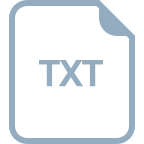
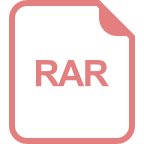
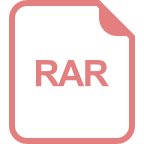
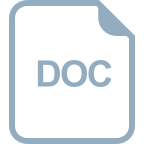
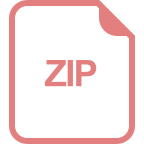
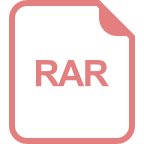
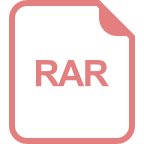
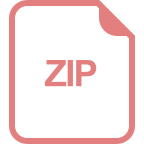
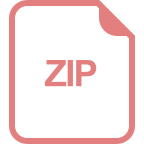
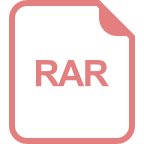
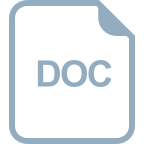
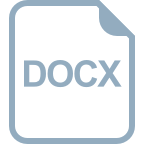