用C语言模拟实现先来先服务、短作业优先、优先级和时间片轮转等操作系统中的进程调度算法。
时间: 2024-02-04 16:03:41 浏览: 24
好的,我可以为您解答这个问题。
首先,需要明确一下进程调度算法的概念。进程调度算法是操作系统用来决定哪个进程可以获得 CPU 时间片的一种策略。常见的调度算法有先来先服务、短作业优先、优先级和时间片轮转等。
下面,我将分别介绍这些调度算法在 C 语言中的模拟实现。
1. 先来先服务调度算法
先来先服务调度算法是最简单的调度算法,它按照进程到达的先后顺序进行调度。实现该算法的代码如下:
```c
#include <stdio.h>
int main() {
int n, i, j;
float bt[20], wt[20], tat[20], avwt = 0, avtat = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time for each process:\n");
for(i = 0; i < n; i++) {
printf("Process %d: ", i + 1);
scanf("%f", &bt[i]);
}
wt[0] = 0;
for(i = 1; i < n; i++) {
wt[i] = 0;
for(j = 0; j < i; j++)
wt[i] += bt[j];
}
printf("\nProcess\t Burst Time\t Waiting Time\t Turnaround Time");
for(i = 0; i < n; i++) {
tat[i] = bt[i] + wt[i];
avwt += wt[i];
avtat += tat[i];
printf("\n %d\t\t%5.2f\t\t%5.2f\t\t%5.2f", i + 1, bt[i], wt[i], tat[i]);
}
avwt /= i;
avtat /= i;
printf("\n\nAverage Waiting Time: %5.2f", avwt);
printf("\nAverage Turnaround Time: %5.2f", avtat);
return 0;
}
```
2. 短作业优先调度算法
短作业优先调度算法是按照进程的执行时间长短来进行调度的。实现该算法的代码如下:
```c
#include <stdio.h>
int main() {
int n, i, j, pos, temp;
float bt[20], wt[20], tat[20], avwt = 0, avtat = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time for each process:\n");
for(i = 0; i < n; i++) {
printf("Process %d: ", i + 1);
scanf("%f", &bt[i]);
}
for(i = 0; i < n; i++) {
pos = i;
for(j = i + 1; j < n; j++) {
if(bt[j] < bt[pos])
pos = j;
}
temp = bt[i];
bt[i] = bt[pos];
bt[pos] = temp;
}
wt[0] = 0;
for(i = 1; i < n; i++) {
wt[i] = 0;
for(j = 0; j < i; j++)
wt[i] += bt[j];
}
printf("\nProcess\t Burst Time\t Waiting Time\t Turnaround Time");
for(i = 0; i < n; i++) {
tat[i] = bt[i] + wt[i];
avwt += wt[i];
avtat += tat[i];
printf("\n %d\t\t%5.2f\t\t%5.2f\t\t%5.2f", i + 1, bt[i], wt[i], tat[i]);
}
avwt /= i;
avtat /= i;
printf("\n\nAverage Waiting Time: %5.2f", avwt);
printf("\nAverage Turnaround Time: %5.2f", avtat);
return 0;
}
```
3. 优先级调度算法
优先级调度算法是按照进程的优先级来进行调度的。实现该算法的代码如下:
```c
#include <stdio.h>
int main() {
int n, i, j, pos, temp, priority[20];
float bt[20], wt[20], tat[20], avwt = 0, avtat = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time and priority for each process:\n");
for(i = 0; i < n; i++) {
printf("Process %d: ", i + 1);
scanf("%f %d", &bt[i], &priority[i]);
}
for(i = 0; i < n; i++) {
pos = i;
for(j = i + 1; j < n; j++) {
if(priority[j] < priority[pos])
pos = j;
}
temp = priority[i];
priority[i] = priority[pos];
priority[pos] = temp;
temp = bt[i];
bt[i] = bt[pos];
bt[pos] = temp;
}
wt[0] = 0;
for(i = 1; i < n; i++) {
wt[i] = 0;
for(j = 0; j < i; j++)
wt[i] += bt[j];
}
printf("\nProcess\t Burst Time\t Waiting Time\t Turnaround Time");
for(i = 0; i < n; i++) {
tat[i] = bt[i] + wt[i];
avwt += wt[i];
avtat += tat[i];
printf("\n %d\t\t%5.2f\t\t%5.2f\t\t%5.2f", i + 1, bt[i], wt[i], tat[i]);
}
avwt /= i;
avtat /= i;
printf("\n\nAverage Waiting Time: %5.2f", avwt);
printf("\nAverage Turnaround Time: %5.2f", avtat);
return 0;
}
```
4. 时间片轮转调度算法
时间片轮转调度算法是按照时间片的大小来分配 CPU 时间的。实现该算法的代码如下:
```c
#include <stdio.h>
int main() {
int n, i, j, time_quantum;
float bt[20], rem_bt[20], wt[20], tat[20], avwt = 0, avtat = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time for each process:\n");
for(i = 0; i < n; i++) {
printf("Process %d: ", i + 1);
scanf("%f", &bt[i]);
rem_bt[i] = bt[i];
}
printf("Enter the time quantum: ");
scanf("%d", &time_quantum);
int t = 0;
while(1) {
int done = 1;
for(i = 0; i < n; i++) {
if(rem_bt[i] > 0) {
done = 0;
if(rem_bt[i] > time_quantum) {
t += time_quantum;
rem_bt[i] -= time_quantum;
} else {
t += rem_bt[i];
wt[i] = t - bt[i];
rem_bt[i] = 0;
}
}
}
if(done == 1) break;
}
for(i = 0; i < n; i++) {
tat[i] = bt[i] + wt[i];
avwt += wt[i];
avtat += tat[i];
}
avwt /= i;
avtat /= i;
printf("\n\nAverage Waiting Time: %5.2f", avwt);
printf("\nAverage Turnaround Time: %5.2f", avtat);
return 0;
}
```
以上就是 C 语言模拟实现先来先服务、短作业优先、优先级和时间片轮转等操作系统中的进程调度算法的代码实现。
相关推荐
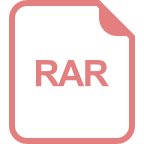














