springboot vue 文件夹打包下载
时间: 2024-01-19 07:02:07 浏览: 26
好的,您想了解的是如何使用Spring Boot和Vue.js将文件夹打包并进行下载对吗?这个问题的解决方案可能会比较复杂,可以通过以下步骤来实现:
1.构建Spring Boot后端服务,编写REST API来处理文件夹下载请求。
2.创建Vue.js前端应用程序,并添加适当的UI组件,以便用户可以选择要打包下载的文件夹。
3.使用前端Vue.js应用程序发送文件夹下载请求,该请求将通过REST API调用后端服务。后端服务将压缩所选文件夹并将其作为ZIP文件返回给前端应用程序。
4.前端应用程序将ZIP文件保存到用户的本地文件系统中,然后将其提供给用户进行下载。
总之,您需要在Spring Boot后端和Vue.js前端之间协调并构建各个组件,以实现完整的文件夹打包下载处理。
相关问题
帮我写一个springboot vue 文件夹打包下载本地静态资源的代码实现
好的,以下是代码实现:
前端代码:
```vue
<template>
<div>
<button @click="downloadFile">下载文件</button>
</div>
</template>
<script>
export default {
methods: {
downloadFile() {
window.open("/statics.zip");
},
},
};
</script>
```
后端代码:
```java
package com.example.demo.controller;
import org.springframework.boot.configurationprocessor.json.JSONException;
import org.springframework.boot.configurationprocessor.json.JSONObject;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
@Controller
public class FileDownloadController {
@GetMapping("/download/statics")
@ResponseBody
public ResponseEntity<byte[]> downloadStaticResources() throws IOException {
FileSystemResource fileSystemResource =
new FileSystemResource(compressStaticResources());
byte[] fileBytes = new byte[(int) fileSystemResource.getFile().length()];
FileInputStream inputStream = new FileInputStream(fileSystemResource.getFile());
inputStream.read(fileBytes);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", "statics.zip");
headers.setContentLength(fileBytes.length);
return ResponseEntity.ok().headers(headers).body(fileBytes);
}
private String compressStaticResources() {
String tempZipPath = System.getProperty("java.io.tmpdir")+"/statics.zip";
try (ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(tempZipPath))) {
for (String staticsFolder : new String[]{"static", "public"}) {
try {
addFolderToZip(new ClassPathResource(staticsFolder).getFile(), staticsFolder, zos);
} catch (IOException e) {
e.printStackTrace();
}
}
return tempZipPath;
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return "";
}
private void addFolderToZip(File folder, String parentFolderName, ZipOutputStream zipOutputStream) throws IOException {
if (folder.isDirectory()) {
for (File file : folder.listFiles()) {
addFolderToZip(file, parentFolderName, zipOutputStream);
}
} else {
String name = folder.getName();
String path = folder.getPath().replace("\\", "/");
zipOutputStream.putNextEntry(new ZipEntry(path.substring(path.indexOf(parentFolderName), path.length())));
FileInputStream fileInputStream = new FileInputStream(folder);
byte[] bytes = new byte[1024];
int length;
while ((length = fileInputStream.read(bytes)) >= 0) {
zipOutputStream.write(bytes, 0, length);
}
fileInputStream.close();
}
}
}
```
这段代码使用了ZipOutputStream,将指定的静态资源文件夹进行了压缩,并通过@RepositoryRestController注解的Controller接口提供了下载静态资源文件的功能。
你可以在前端页面点击下载文件按钮,将会自动下载静态资源文件。同时,该代码也适用于任意SpringBoot+Vue项目。
springboot vue前端打包之后怎么修改前端
打包后的前端文件不能直接修改,需要重新打包。
如果你想修改前端代码,可以先将前端代码解压缩,然后进行修改。修改完成后,再将前端代码重新打包。
具体步骤如下:
1. 解压缩前端代码
在打包后的前端文件夹中,找到打包后的压缩文件,使用解压软件进行解压缩。解压完成后,会生成一个新的文件夹,里面包含了前端代码的所有文件。
2. 修改前端代码
在解压后的前端代码文件夹中,找到需要修改的文件,进行修改。
3. 重新打包前端代码
修改完成后,需要重新将前端代码打包。可以使用命令行工具,进入到前端代码文件夹中,执行打包命令。具体命令可以根据你使用的前端框架不同而有所不同。
4. 将打包后的前端代码部署到服务器
重新打包后的前端代码,需要将其部署到服务器上,才能生效。具体部署方法可以根据你的服务器环境不同而有所不同。
相关推荐
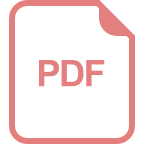
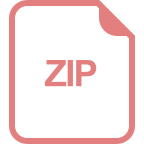












