#include <iostream> #include <string> using namespace std; /* run this program using the console pauser or add your own getch, system("pause") or input loop */ class Student { private: string name; //姓名 string id; //学号 double cpp_score; //C++成绩 double math_score; //数学成绩 double Eng_score; //英语成绩 public: //此处定义构造函数 //--------------------------------------- void set(string n,string i,double c,double m,double e);//数据输入 void show();//数据输出到屏幕上 //此处定义析构函数 //--------------------------------------- }; void Student::set(string n, string i, double c, double m, double e) { name = n; id = i; cpp_score = c; math_score = m; Eng_score = e; } void Student::show() { cout << "姓名:" << name << endl; cout << "ID:" << id << endl; cout << "C++成绩:" << cpp_score << endl; cout << "数学成绩:" << math_score << endl; cout << "英语成绩:" << Eng_score << endl; } int main(int argc, char** argv) { string n; string i; double c; double m; double e; cin >> n >> i >> c >> m >> e; Student s1; Student s2(n, i, c, m, e); s1.show(); s2.show(); return 0; }
时间: 2024-03-10 20:50:08 浏览: 81
这段代码存在一些问题,无法正常运行,具体问题包括:
1. 在`Student`类中,没有定义构造函数和析构函数。
2. 在`main`函数中,虽然定义了两个`Student`类型的对象`s1`和`s2`,但是只有`s2`通过构造函数传递了参数,而`s1`仍然是一个未初始化的对象,会导致输出的内容不符合实际。
下面是修改后的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string name; //姓名
string id; //学号
double cpp_score; //C++成绩
double math_score; //数学成绩
double Eng_score; //英语成绩
public:
//构造函数
Student() {} //默认构造函数
Student(string n, string i, double c, double m, double e) : name(n), id(i), cpp_score(c), math_score(m), Eng_score(e) {}
//析构函数
~Student() {}
void set(string n, string i, double c, double m, double e);//数据输入
void show();//数据输出到屏幕上
};
void Student::set(string n, string i, double c, double m, double e) {
name = n;
id = i;
cpp_score = c;
math_score = m;
Eng_score = e;
}
void Student::show() {
cout << "姓名:" << name << endl;
cout << "ID:" << id << endl;
cout << "C++成绩:" << cpp_score << endl;
cout << "数学成绩:" << math_score << endl;
cout << "英语成绩:" << Eng_score << endl;
}
int main() {
string n;
string i;
double c;
double m;
double e;
cin >> n >> i >> c >> m >> e;
Student s1; //定义一个默认构造函数的对象
Student s2(n, i, c, m, e); //定义一个带参数的构造函数的对象
s1.set("Mike", "001", 89, 87, 92); //手动设置s1对象的数据
s1.show();
s2.show();
return 0;
}
```
在修改后的代码中,我们定义了一个默认构造函数和一个带参数的构造函数,同时也定义了析构函数。在`main`函数中,我们创建了两个`Student`类型的对象`s1`和`s2`,并通过构造函数传递参数。同时,我们也手动设置了`s1`对象的数据,保证输出的内容正确。
阅读全文
相关推荐
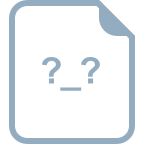
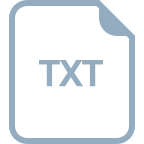
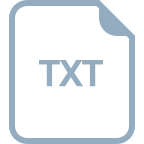
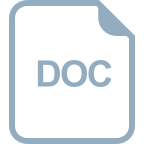
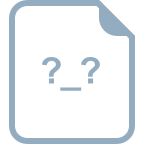
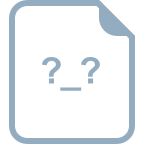
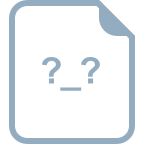
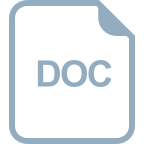
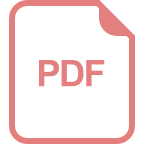
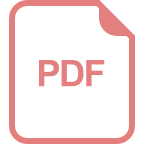
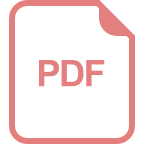
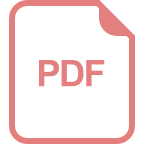
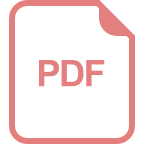
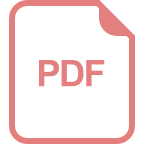
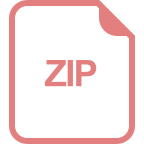
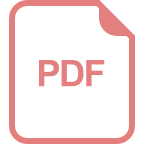
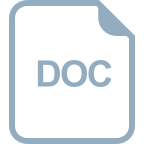