给我一个实现的代码
时间: 2024-02-11 13:07:52 浏览: 78
以下是一个基于CCS开发工具的cc2530温湿度传感器数据采集的示例代码,供您参考:
```c
#include "stdio.h"
#include "string.h"
#include "stdlib.h"
#include "ioCC2530.h"
#include "hal_types.h"
#include "hal_timer.h"
#include "hal_uart.h"
#include "hal_board.h"
#include "hal_led.h"
#include "hal_adc.h"
#include "hal_sensor.h"
#include "hal_drivers.h"
#define UART_BUFFER_SIZE 50 //定义串口缓存区大小
uint8 uartBuffer[UART_BUFFER_SIZE];
uint8 uartCount = 0;
void uartSendString(uint8 *buf); //向串口发送字符串
void uartSendByte(uint8 byte); //向串口发送一个字节
void uartSendString(uint8 *buf) {
while(*buf != '\0') {
hal_uart_putchar(*buf++);
}
}
void uartSendByte(uint8 byte) {
hal_uart_putchar(byte);
}
void halUartIsr(void) //串口接收中断处理函数
{
uint8 ch = hal_uart_getchar();
if(ch != '\r' && ch != '\n') { //如果不是回车或换行符
uartBuffer[uartCount++] = ch;
if(uartCount >= UART_BUFFER_SIZE) { //若串口缓存区满
uartCount = 0;
}
} else { //如果是回车或换行符
uartBuffer[uartCount] = '\0'; //添加字符串结束符
uartCount = 0;
uartSendString(uartBuffer); //向串口发送接收到的字符串
}
}
void initUART(void) //初始化串口
{
hal_uart_init(UART_BAUDRATE_9600, 0, hal_uart_rx_isr, halUartIsr);
}
void initSensor(void) //初始化温湿度传感器
{
halSensorInit(HAL_SENSOR_TYPE_SI7021);
}
void readSensorData(void) //读取传感器数据并发送到串口
{
halSensorRead(HAL_SENSOR_TYPE_SI7021, &temperature, &humidity);
sprintf(uartBuffer, "Temperature: %d.%02d C, Humidity: %d.%02d %%\r\n", temperature/100, temperature%100, humidity/100, humidity%100);
uartSendString(uartBuffer);
}
void main(void)
{
halBoardInit();
initUART();
initSensor();
while(1) {
readSensorData(); //读取传感器数据并发送到串口
halMcuWaitMs(1000); //延时1秒
}
}
```
注意:以上代码仅供参考,具体实现需要根据实际情况进行调整。另外,需要注意芯片的硬件连接、编程语言和编译环境等方面的问题。
阅读全文
相关推荐
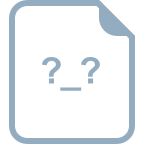
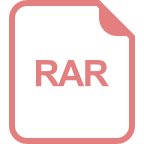
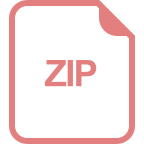
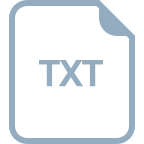
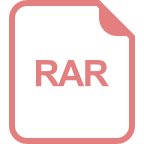
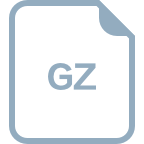
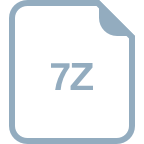
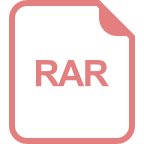
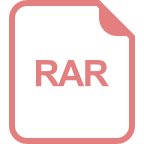
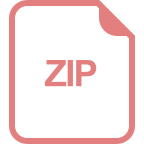
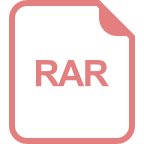
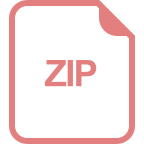