C# EMgucv 机器学习 图像分类的训练与测试
时间: 2023-08-29 11:04:46 浏览: 279
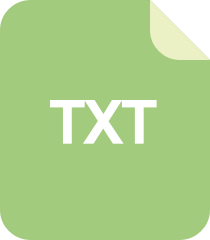
C#下配置OPENCV

以下是使用EMGU.CV进行图像分类训练和测试的C#代码示例:
```csharp
using Emgu.CV;
using Emgu.CV.CvEnum;
using Emgu.CV.ML;
using Emgu.CV.Structure;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ImageClassification
{
class Program
{
static void Main(string[] args)
{
// Load the training data
Matrix<float> trainingData = new Matrix<float>(new float[,]
{
{ 100, 200 },
{ 150, 250 },
{ 200, 300 },
{ 250, 350 },
{ 300, 400 },
{ 350, 450 },
{ 400, 500 },
{ 450, 550 }
});
// Load the labels for the training data
Matrix<float> labels = new Matrix<float>(new float[,]
{
{ 0 },
{ 0 },
{ 0 },
{ 0 },
{ 1 },
{ 1 },
{ 1 },
{ 1 }
});
// Initialize the SVM model
SVM model = new SVM();
// Set the SVM parameters
var svmParams = new MCvSVMParams()
{
KernelType = SVM_KERNEL_TYPE.LINEAR,
C = 1,
Gamma = 0.5,
TermCrit = new MCvTermCriteria(100, 0.00001)
};
// Train the SVM model
model.Train(trainingData, labels, null, null, svmParams);
// Load the test data
Matrix<float> testData = new Matrix<float>(new float[,]
{
{ 125, 225 },
{ 225, 325 },
{ 325, 425 },
{ 425, 525 }
});
// Predict the labels for the test data
Matrix<float> predictedLabels = new Matrix<float>(testData.Rows, 1);
model.Predict(testData, predictedLabels);
// Output the predicted labels
Console.WriteLine("Predicted Labels:");
for (int i = 0; i < predictedLabels.Rows; i++)
{
Console.WriteLine(predictedLabels[i, 0]);
}
// Wait for user input
Console.ReadLine();
}
}
}
```
上面的代码演示了如何使用EMGU.CV进行图像分类的训练和测试。在此示例中,我们使用了一个简单的线性SVM模型来预测输入数据的标签。我们首先加载训练数据和标签,然后使用SVM.Train方法训练模型。一旦我们训练好了模型,我们就可以加载测试数据,并使用SVM.Predict方法预测测试数据的标签。最后,我们可以输出预测的标签以进行检查。
需要注意的是,此示例是基于简单的数值输入数据进行训练和测试的,实际上在图像分类中需要使用更复杂的特征提取和预处理方法,以及更大的数据集来训练和测试模型。
阅读全文
相关推荐
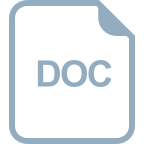
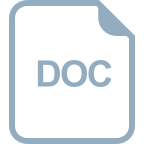


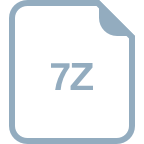
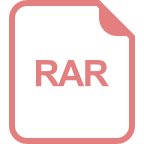
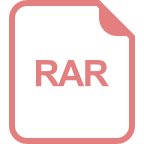
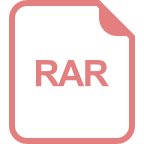
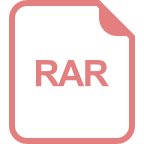
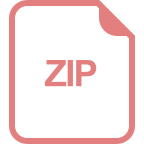
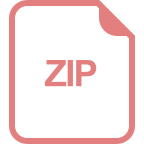
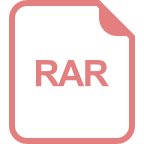
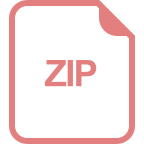
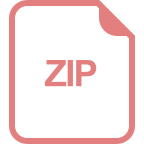
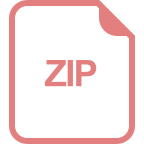
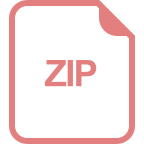
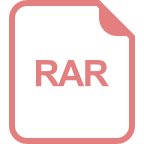
