c语言实现链表的插入 并对比链表插入前后的序列
时间: 2023-07-12 11:20:41 浏览: 96
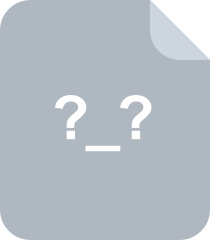
c语言实现链表的操作
好的,针对您的问题,我可以给出以下示例代码来实现链表的插入操作:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void insert(struct Node** head, int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
struct Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
void display(struct Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
struct Node* head = NULL;
// 插入 1, 2, 3, 4
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
insert(&head, 4);
printf("插入前:");
display(head);
// 插入 5
insert(&head, 5);
printf("插入后:");
display(head);
return 0;
}
```
在上述代码中,我们首先定义了一个 `Node` 结构体来表示链表中的每个节点,其中包括一个整型数据 `data` 和一个指向下一个节点的指针 `next`。
然后,我们实现了 `insert` 函数,该函数接收一个指向链表头部指针的指针和要插入的数据。该函数先创建一个新节点 `newNode`,并将数据存储在其中,然后将其指针指向 `NULL`。接着,如果链表为空,则将其设置为 `head`;否则,遍历链表并将新节点添加到链表末尾。
最后,我们在 `main` 函数中演示了如何使用 `insert` 函数插入数据,并打印出了插入前后的链表序列。
您可以对比插入前后的链表序列,发现链表已经成功插入新节点。
阅读全文
相关推荐
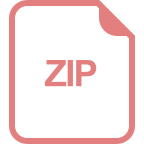
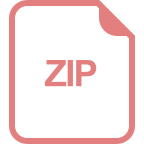
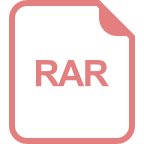
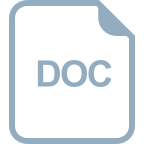
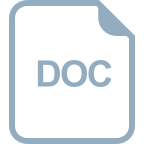
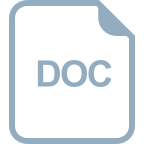
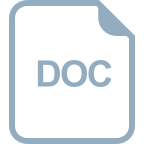
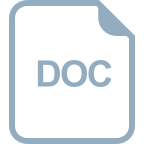
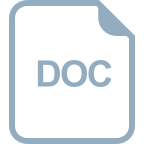
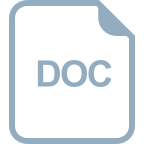
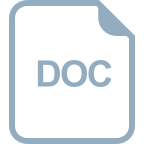
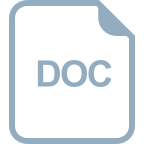
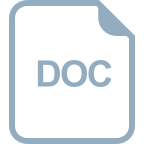
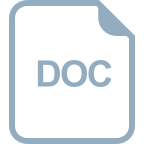

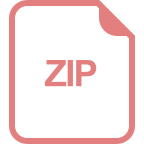