运用嵌套if结构和switch结构 多重if选择结构 实现商品换购功能
时间: 2024-09-30 19:15:21 浏览: 35
在JavaScript中,你可以使用多重`if`选择结构或`switch`结构来实现商品换购功能。这里我们假设有一个商品列表,每个商品都有原价、折扣价以及是否参与换购活动。以下是一个简单的例子:
```javascript
let product = {
name: 'Product A',
originalPrice: 100,
discountedPrice: null, // 可能为null表示未打折
onSale: false, // 是否参与换购
};
function checkExchange(product) {
if (product.onSale) { // 检查商品是否在换购活动
if (product.discountedPrice === null) { // 如果没有打折价格,则计算折扣价
product.discountedPrice = calculateDiscountedPrice(product.originalPrice); // 假设calculateDiscountedPrice是个函数
}
console.log(`You can exchange ${product.name} for the discounted price of $${product.discountedPrice}`);
} else {
console.log(`${product.name} is not on sale.`);
}
}
// 示例:如果商品A在换购活动中并且原价大于某个阈值,有额外优惠
function calculateDiscountedPrice(originalPrice) {
if (originalPrice > 50) {
return originalPrice * 0.8; // 给予8折优惠
} else {
return originalPrice;
}
}
checkExchange(product);
```
在这个例子中,`checkExchange`函数首先检查商品是否在换购活动中,然后根据情况决定是否计算折扣价并输出相应的信息。
如果你想用`switch`结构来实现相同的功能,可能需要转换逻辑结构,因为`switch`主要针对有限的条件分支:
```javascript
function checkExchangeWithSwitch(product) {
switch (true) {
case product.onSale && product.discountedPrice === null:
product.discountedPrice = calculateDiscountedPrice(product.originalPrice);
break;
case !product.onSale:
console.log(`${product.name} is not on sale.`);
break;
default:
console.log('Invalid condition.');
}
console.log(`You can exchange ${product.name} for the discounted price of $${product.discountedPrice}`);
}
checkExchangeWithSwitch(product);
```
但请注意,`switch`在这里并不是最佳选择,因为它不如`if...else`链更直观,特别是在处理多个复杂的逻辑条件时。
阅读全文
相关推荐
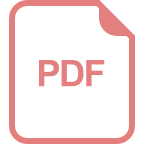
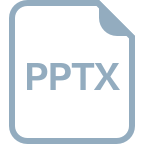
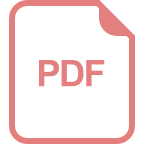















