VS2019 C++ 通过 filesystem 对外部普通的配置文件中字符串读写功能的实现,不需要键值对的实现方法并可以读取指定行的信息
时间: 2024-10-22 14:09:42 浏览: 17
在Visual Studio 2019的C++中,如果你想要通过`filesystem`库直接读写外部普通文本配置文件(如`.txt`),而无需使用键值对,你可以使用文件流(fstream)来操作。以下是基本的步骤:
1. 包含头文件:
```cpp
#include <iostream>
#include <filesystem> // for filesystem operations
```
2. 创建文件读写函数:
```cpp
std::string readLineFromFile(const std::string& filePath, size_t lineNumber) {
std::ifstream file(filePath);
if (!file.is_open()) {
throw std::runtime_error("Failed to open the file.");
}
std::streampos position = lineNumber - 1; // Subtract one because file indexing starts from 0
file.seekg(position, std::ios_base::beg); // Seek to the specified line
std::string line;
std::getline(file, line);
file.close();
return line;
}
void writeLineToFile(const std::string& filePath, const std::string& content, size_t lineNumber) {
std::ofstream file(filePath, std::ios::app); // 'a' for appending
if (!file.is_open()) {
throw std::runtime_error("Failed to open the file for writing.");
}
file << "\n" << content; // Add newline before writing
file.seekp(lineNumber * '\n', std::ios_base::cur); // Go back to the start of the line
file << content; // Write the content
file.close();
}
```
3. 使用示例:
```cpp
int main() {
try {
std::string configFile = "config.txt";
std::string lineToRead = readLineFromFile(configFile, 2); // Read second line
std::cout << "Line 2 content: " << lineToRead << std::endl;
std::string newLine = "New configuration value";
writeLineToFile(configFile, newLine, 5); // Append to line 5
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
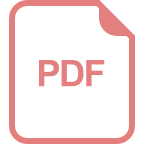
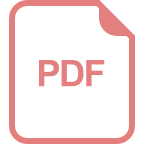
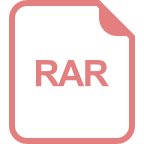



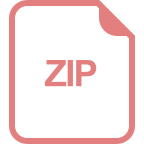
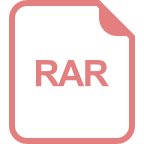
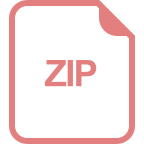
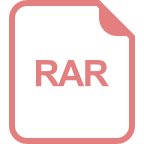
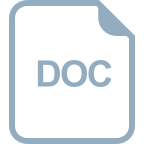
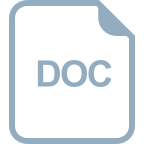
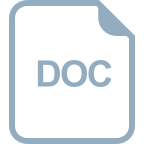
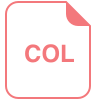
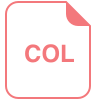
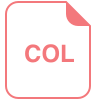
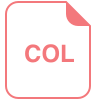
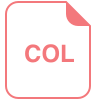
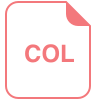