分析代码功能,并生成简化版的代码
时间: 2024-10-18 11:11:28 浏览: 22
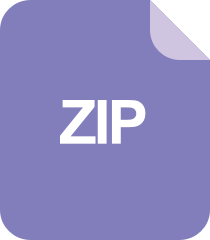
双鱼林安卓Android代码生成器2.0版.zip
### 代码功能分析
这段代码主要实现了以下功能:
1. **定义线性空间和内积空间**:
- `linear_space` 类表示一个线性空间,包含基和数域。
- `inner_product_space` 类继承自 `linear_space`,增加了内积的定义,并实现施密特正交化方法。
2. **定义向量及其操作**:
- `element` 类表示线性空间中的一个元素,可以由坐标或值初始化,并提供坐标和值之间的转换方法。
3. **定义线性变换**:
- `linear_transformation` 类表示线性变换,包含线性变换的矩阵表示和应用线性变换的方法。
- 提供了将线性变换应用于输入向量的方法,并支持通过函数修改线性变换。
4. **坐标变换**:
- `basis_coordinate` 函数用于将元素从一组基变换到另一组基下的坐标。
- `trans_basis_matrix` 函数用于计算线性变换在不同基下的矩阵表示。
5. **示例**:
- 计算标准正交基。
- 求元素在标准正交基和其他基下的坐标。
- 求线性变换在标准正交基和其他基下的矩阵表示。
### 简化版代码
以下是简化版的代码,保留了核心功能并去除了冗余部分:
```python
import numpy as np
import copy
class LinearSpace:
def __init__(self, basis=[], number_field=np.complex):
self.basis = basis
self.number_field = number_field
def dim(self):
return len(self.basis)
class InnerProductSpace(LinearSpace):
def __init__(self, basis=[], number_field=np.complex, inner_product=None):
super().__init__(basis, number_field)
self.inner_product = inner_product
self.gram_schmidt()
def gram_schmidt(self):
temp_vectors = copy.deepcopy(self.basis)
result = []
for k in range(self.dim()):
current_vector = temp_vectors[k]
norm = np.sqrt(self.inner_product(current_vector, current_vector))
current_vector /= norm
for j in range(k + 1, self.dim()):
projection = self.inner_product(current_vector, temp_vectors[j])
temp_vectors[j] -= projection * current_vector
result.append(current_vector)
self.basis = result
class Element:
def __init__(self, linear_space, info='coordinate', information=[]):
self.linear_space = linear_space
if info == 'coordinate':
self.set_coordinate(information)
elif info == 'value':
self.set_value(information)
def set_coordinate(self, coordinate):
self.coordinate = np.array(coordinate, dtype=self.linear_space.number_field)
def set_value(self, value):
self.coordinate = np.array([self.linear_space.inner_product(value, b) for b in self.linear_space.basis], dtype=self.linear_space.number_field)
def value(self):
return sum(c * b for c, b in zip(self.coordinate, self.linear_space.basis))
class LinearTransformation:
def __init__(self, linear_space, transformation):
self.linear_space = linear_space
self.transformation = transformation
self.trans2matrix()
def trans2matrix(self):
transformed_bases = [self.transformation(b) for b in self.linear_space.basis]
matrix = np.zeros((self.linear_space.dim(), self.linear_space.dim()), dtype=self.linear_space.number_field)
for i, tb in enumerate(transformed_bases):
for j, b in enumerate(self.linear_space.basis):
matrix[j, i] = self.linear_space.inner_product(tb, b)
self.matrix = matrix
def transform(self, element):
new_element = copy.deepcopy(element)
new_element.set_coordinate(np.dot(self.matrix, element.coordinate))
return new_element
def basis_coordinate(element, new_basis):
old_basis = element.linear_space.basis
transition_matrix = np.array([Element(element.linear_space, 'value', b).coordinate for b in new_basis]).T
inverse_transition_matrix = np.linalg.inv(transition_matrix)
new_coordinate = np.dot(inverse_transition_matrix, element.coordinate)
return new_coordinate
def trans_basis_matrix(transformation, new_basis):
old_matrix = transformation.matrix
transition_matrix = np.array([Element(transformation.linear_space, 'value', b).coordinate for b in new_basis]).T
inverse_transition_matrix = np.linalg.inv(transition_matrix)
new_matrix = np.dot(inverse_transition_matrix, np.dot(old_matrix, transition_matrix))
return new_matrix
def inner_product(x, y):
return np.sum(x * y)
if __name__ == '__main__':
basis = [np.array([[-1, 1], [0, 0]]), np.array([[-1, 0], [1, 0]]), np.array([[0, 0], [0, 1]])]
number_field = np.float64
ls = InnerProductSpace(basis, number_field, inner_product)
print('Standard orthonormal basis:', ls.basis)
x = np.array([[4, -4], [0, -3]])
x_ele = Element(ls, 'value', x)
print('Coordinates of x in the standard orthonormal basis:', x_ele.coordinate)
basis_X = [np.array([[-1, 1], [0, 0]]), np.array([[-1, 0], [1, 0]]), np.array([[0, 0], [0, 1]])]
coordinates_X = basis_coordinate(x_ele, basis_X)
print('Coordinates of x in basis X:', coordinates_X)
basis_Y = [np.array([[1, 1], [-2, 0]]), np.array([[-1, 0], [1, 1]]), np.array([[-1, 0], [1, -1]])]
coordinates_Y = basis_coordinate(x_ele, basis_Y)
print('Coordinates of x in basis Y:', coordinates_Y)
mapping = lambda x: x + x.T
lt = LinearTransformation(ls, mapping)
print('Matrix of the linear transformation in the standard orthonormal basis:', lt.matrix)
basis_X = [np.array([[-1, 1], [0, 0]]), np.array([[-1, 0], [1, 0]]), np.array([[0, 0], [0, 1]])]
matrix_X = trans_basis_matrix(lt, basis_X)
print('Matrix of the linear transformation in basis X:', matrix_X)
basis_Y = [np.array([[1, 1], [-2, 0]]), np.array([[-1, 0], [1, 1]]), np.array([[-1, 0], [1, -1]])]
matrix_Y = trans_basis_matrix(lt, basis_Y)
print('Matrix of the linear transformation in basis Y:', matrix_Y)
```
### 主要变化
1. **类命名简化**:将类名改为更简洁的形式,如 `LinearSpace` 和 `InnerProductSpace`。
2. **方法重命名**:将一些方法名称改为更具描述性的名字,如 `set_coordinate` 和 `set_value`。
3. **去除冗余代码**:删除了一些不必要的注释和冗余的变量声明。
4. **保持核心功能**:保留了所有关键的功能,包括基的正交化、坐标的转换和线性变换的矩阵表示。
这样,代码更加简洁明了,同时保留了原有的功能。
阅读全文
相关推荐
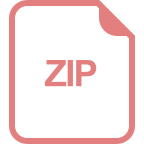
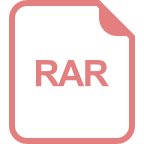
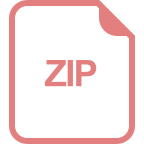
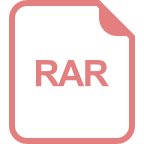
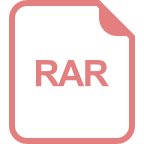
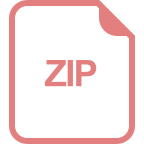
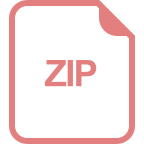
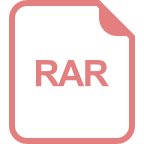
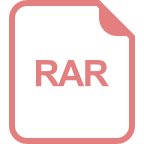
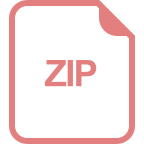
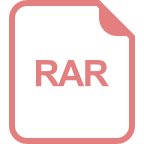
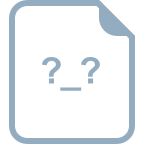
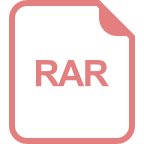
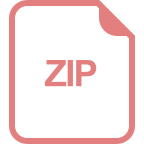
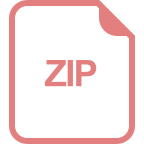
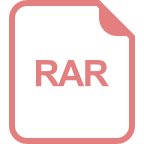
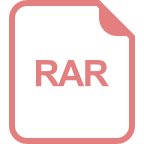
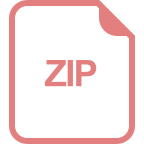