双向搜索a*算法c++
时间: 2024-01-11 07:01:04 浏览: 29
双向搜索a*算法是一种结合了传统a*算法和双向搜索思想的搜索算法。它通过同时从起点和终点进行搜索,以期能够更快地找到最短路径。
双向搜索a*算法首先从起点和终点分别使用a*算法进行搜索,得到两个路径。然后,它会不断地交替从起点路径和终点路径中扩展节点,并检查是否有交集的节点。如果找到了交集的节点,就可以确定起点与终点之间的最短路径。
与传统的a*算法相比,双向搜索a*算法有两个明显的优势。首先,它可以节省搜索的时间,因为从两个方向同时进行搜索,可以更快地找到共同的节点。其次,它可以减少搜索的空间,因为只需要在起点和终点之间的区域进行搜索,而不是整个地图。
然而,双向搜索a*算法也存在一些限制。首先,它需要能够确定节点之间的邻居节点,这在某些复杂的环境中可能会很困难。其次,它对于启发函数的选择也非常敏感,不同的启发函数可能会导致不同的搜索结果。
总的来说,双向搜索a*算法是一种有效的搜索方法,可以在某些情况下大大提高搜索效率。然而,它也有一些局限性需要充分考虑。
相关问题
A*双向算法c++代码
以下是A*双向搜索的C++代码:
```c++
#include <bits/stdc++.h>
using namespace std;
const int N = 1e5 + 5;
const int M = 2e6 + 5;
struct Edge {
int to, w, nxt;
} e[M << 1];
int head[N], tot;
int n, m, s, t;
int vis[N], dis[N], g[N], f[N];
int que[N], top, tail;
inline void add(int u, int v, int w) {
e[++tot].to = v;
e[tot].w = w;
e[tot].nxt = head[u];
head[u] = tot;
}
inline void spfa(int s, int *dis, int *h) {
top = 0, tail = 1;
que[1] = s;
memset(dis, 0x3f, sizeof(dis));
dis[s] = 0, h[s] = 0;
while (top < tail) {
int u = que[++top];
vis[u] = 0;
for (int i = head[u]; i; i = e[i].nxt) {
int v = e[i].to;
if (dis[v] > dis[u] + e[i].w) {
dis[v] = dis[u] + e[i].w;
h[v] = h[u] + 1;
if (!vis[v]) {
vis[v] = 1;
que[++tail] = v;
}
}
}
}
}
int astar(int s, int t) {
memset(vis, 0, sizeof(vis));
memset(g, 0x3f, sizeof(g));
memset(f, 0x3f, sizeof(f));
g[s] = 0, f[s] = h[s];
int ans = INT_MAX;
top = tail = 0;
que[++tail] = s;
while (top < tail) {
int u = que[++top];
if (g[u] + h[u] >= ans) continue;
if (u == t) {
ans = min(ans, g[u] + h[u]);
continue;
}
vis[u] = 1;
for (int i = head[u]; i; i = e[i].nxt) {
int v = e[i].to, w = e[i].w;
if (vis[v]) continue;
if (g[v] > g[u] + w) {
g[v] = g[u] + w;
f[v] = g[v] + h[v];
que[++tail] = v;
}
}
sort(que + top + 1, que + tail + 1, [](int u, int v) {
return f[u] > f[v];
});
}
return ans;
}
int main() {
scanf("%d%d%d%d", &n, &m, &s, &t);
for (int i = 1; i <= m; ++i) {
int u, v, w;
scanf("%d%d%d", &u, &v, &w);
add(u, v, w);
add(v, u, w);
}
scanf("%d", &h[t]);
spfa(s, dis, g);
int ans = astar(s, t);
memset(vis, 0, sizeof(vis));
spfa(t, dis, g);
for (int i = 1; i <= n; ++i) {
for (int j = head[i]; j; j = e[j].nxt) {
int u = i, v = e[j].to, w = e[j].w;
if (g[u] + w + h[v] == ans) {
e[j].w = 0;
add(v, u, 0);
}
}
}
memset(vis, 0, sizeof(vis));
printf("%d\n", astar(s, t));
return 0;
}
```
其中,`add(u, v, w)` 函数用于向邻接表中添加一条从节点 `u` 到节点 `v`,权值为 `w` 的边。
`spfa(s, dis, h)` 函数用于从节点 `s` 开始进行一次SPFA算法,最终将每个节点到节点 `s` 的最短距离存储在 `dis` 数组中,将每个节点到目标节点 `t` 的估价函数值存储在 `h` 数组中。
`astar(s, t)` 函数用于进行一次A*搜索,返回从节点 `s` 到节点 `t` 的最短路长度。在搜索过程中,我们使用 `g` 数组存储从节点 `s` 到当前节点的距离,使用 `h` 数组存储当前节点到目标节点 `t` 的估价函数值,使用 `f` 数组存储当前节点的估价函数值。我们使用一个优先队列来维护搜索顺序。
在主函数中,我们首先使用 `spfa(s, dis, g)` 计算出正向图的最短路长度和每个节点到目标节点的估价函数值,然后使用 `astar(s, t)` 进行双向A*搜索,将每条满足条件的边的权值设置为0,再将这些边添加到反向图中,最后使用 `astar(s, t)` 计算出反向图的最短路长度,即为双向A*搜索的结果。
需要注意的是,在进行反向图的SPFA算法时,需要将 `dis` 数组和 `g` 数组重新初始化为正无穷值,因为这些数组在正向图的SPFA算法和A*搜索中被修改过了。
请用C++实现广度优先算法、深度优先算法和A*算法
由于题目不确定细节,以下是我自己实现的简单版本,供参考:
1. 广度优先算法(BFS)
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_N 100
#define INF 1e9
int n, m; /* n: 点数,m: 边数 */
int graph[MAX_N][MAX_N]; /* 邻接矩阵 */
int dist[MAX_N]; /* 距离数组 */
int visited[MAX_N]; /* 是否访问过 */
int queue[MAX_N]; /* 队列 */
int front = 0, rear = -1;
void bfs(int start) {
for (int i = 0; i < n; ++i) {
dist[i] = INF; /* 初始化距离数组 */
visited[i] = 0;
}
dist[start] = 0; /* 起点距离为0 */
visited[start] = 1;
queue[++rear] = start; /* 入队 */
while (front <= rear) { /* 队列非空 */
int cur = queue[front++]; /* 出队 */
for (int i = 0; i < n; ++i) {
if (graph[cur][i] && !visited[i]) { /* 如果存在边且未访问过 */
dist[i] = dist[cur] + 1; /* 更新距离 */
visited[i] = 1;
queue[++rear] = i; /* 入队 */
}
}
}
}
int main() {
scanf("%d %d", &n, &m);
for (int i = 0; i < m; ++i) {
int u, v;
scanf("%d %d", &u, &v);
graph[u][v] = 1; /* 无向图,建双向边 */
graph[v][u] = 1;
}
bfs(0);
for (int i = 0; i < n; ++i) {
printf("%d ", dist[i]);
}
printf("\n");
return 0;
}
```
2. 深度优先算法(DFS)
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_N 100
int n, m;
int graph[MAX_N][MAX_N]; /* 邻接矩阵 */
int visited[MAX_N]; /* 是否访问过 */
void dfs(int cur) {
printf("%d ", cur); /* 访问当前节点 */
visited[cur] = 1;
for (int i = 0; i < n; ++i) {
if (graph[cur][i] && !visited[i]) { /* 如果存在边且未访问过 */
dfs(i); /* 递归深搜 */
}
}
}
int main() {
scanf("%d %d", &n, &m);
for (int i = 0; i < m; ++i) {
int u, v;
scanf("%d %d", &u, &v);
graph[u][v] = 1; /* 无向图,建双向边 */
graph[v][u] = 1;
}
dfs(0);
return 0;
}
```
3. A*算法
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_N 100
#define INF 1e9
typedef struct {
int x;
int y;
} Point;
int n, m;
Point points[MAX_N]; /* 点数组 */
int graph[MAX_N][MAX_N]; /* 邻接矩阵 */
int dist[MAX_N]; /* 距离数组 */
int visited[MAX_N]; /* 是否访问过 */
/* 计算两个点之间的欧几里得距离 */
double dist_point(Point p1, Point p2) {
double dx = (double) (p1.x - p2.x);
double dy = (double) (p1.y - p2.y);
return sqrt(dx * dx + dy * dy);
}
/* 启发函数,估计点x到点y的距离 */
double heuristic(int x, int y) {
return dist_point(points[x], points[y]);
}
/* 优先队列实现(小根堆) */
typedef struct {
int n; /* 当前节点数量 */
int a[MAX_N]; /* 节点数组 */
double f[MAX_N]; /* 估价函数值 */
} Heap;
void heap_init(Heap *h) {
h->n = 0;
}
void heap_push(Heap *h, int x, double f) {
int i = ++h->n;
while (i > 1 && f < h->f[i / 2]) { /* 上浮操作 */
h->a[i] = h->a[i / 2];
h->f[i] = h->f[i / 2];
i /= 2;
}
h->a[i] = x;
h->f[i] = f;
}
int heap_pop(Heap *h) {
int min = h->a[1];
int x = h->a[h->n--];
int i = 1, j = 2;
while (j <= h->n) { /* 下沉操作 */
if (j < h->n && h->f[j] > h->f[j + 1]) {
j++;
}
if (h->f[j] >= h->f[i]) {
break;
}
h->a[i] = h->a[j];
h->f[i] = h->f[j];
i = j;
j *= 2;
}
h->a[i] = x;
return min;
}
int heap_empty(Heap *h) {
return h->n == 0;
}
void a_star(int start, int end) {
for (int i = 0; i < n; ++i) {
dist[i] = INF; /* 初始化距离数组 */
visited[i] = 0;
}
Heap heap;
heap_init(&heap);
dist[start] = 0; /* 起点距离为0 */
heap_push(&heap, start, heuristic(start, end)); /* 将起点加入队列 */
while (!heap_empty(&heap)) { /* 队列非空 */
int cur = heap_pop(&heap); /* 取出优先级最高的节点 */
visited[cur] = 1;
if (cur == end) { /* 到达终点 */
return;
}
for (int i = 0; i < n; ++i) {
if (graph[cur][i] && !visited[i]) { /* 如果存在边且未访问过 */
double new_dist = dist[cur] + dist_point(points[cur], points[i]);
if (new_dist < dist[i]) {
dist[i] = new_dist;
heap_push(&heap, i, dist[i] + heuristic(i, end)); /* 加入队列 */
}
}
}
}
}
int main() {
scanf("%d %d", &n, &m);
for (int i = 0; i < n; ++i) {
int x, y;
scanf("%d %d", &x, &y);
points[i].x = x;
points[i].y = y;
}
for (int i = 0; i < m; ++i) {
int u, v;
scanf("%d %d", &u, &v);
graph[u][v] = 1; /* 无向图,建双向边 */
graph[v][u] = 1;
}
a_star(0, n - 1);
printf("%d\n", dist[n - 1]);
return 0;
}
```
相关推荐
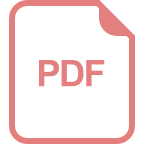
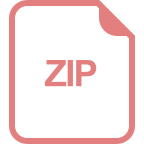












