获取char类型文件路径中的文件名
时间: 2024-05-05 13:19:41 浏览: 13
你可以使用C++中的标准库函数`std::string`和`std::string_view`来获取char类型文件路径中的文件名。具体方法如下:
1. 将char类型的文件路径转换为std::string类型:
```c++
const char* path = "/path/to/file.txt";
std::string path_str(path);
```
2. 使用std::string的find_last_of函数找到最后一个路径分隔符("/")的位置:
```c++
size_t pos = path_str.find_last_of("/");
```
3. 使用std::string_view从std::string中提取文件名:
```c++
std::string_view filename = path_str.substr(pos + 1);
```
这里使用std::string_view可以避免额外的字符串拷贝操作,提高效率。
4. 最后将std::string_view转换为std::string:
```c++
std::string filename_str(filename);
```
如果你只需要文件名的字符数组,可以使用filename_str.c_str()函数获取。
相关问题
matlab 获取char类型文件路径中的文件名
您可以使用Matlab中的`fileparts`函数来获取char类型文件路径中的文件名。
例如,如果您的文件路径为`'C:\Users\UserName\Documents\example.txt'`,则可以使用以下代码来获取文件名:
```
[~, filename, ~] = fileparts('C:\Users\UserName\Documents\example.txt');
```
`filename`变量将包含文件名`'example'`。
win32程序中如何根据文件路径获取文件名?
可以使用Windows API函数PathFindFileName来获取文件路径中的文件名。
具体实现方法如下:
1. 引入Windows.h头文件。
2. 调用PathFindFileName函数,传入文件路径参数,返回文件名指针。
示例代码如下:
```c++
#include <Windows.h>
#include <Shlwapi.h> // 引入Shlwapi库
#pragma comment(lib, "Shlwapi.lib") // 链接Shlwapi库
int main()
{
const char* filePath = "C:\\Users\\xx\\Desktop\\test.txt";
const char* fileName = PathFindFileName(filePath);
printf("File name: %s\n", fileName);
return 0;
}
```
输出结果:
```
File name: test.txt
```
相关推荐
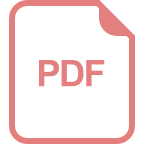
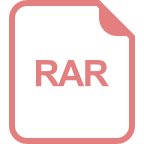
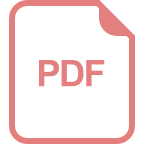












