用Python编写程序用线性回归方程预测GPA分数
时间: 2024-05-06 07:21:40 浏览: 106
可以使用Python中的scikit-learn库来实现线性回归模型,下面是示例代码:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
# 创建X和Y的样本数据
X = np.array([[2.0, 4.0], [3.0, 6.0], [4.0, 8.0], [5.0, 10.0]])
y = np.array([3.0, 4.0, 5.0, 6.0])
# 创建线性回归模型并训练
model = LinearRegression()
model.fit(X, y)
# 使用模型预测新数据的GPA分数
new_data = np.array([[6.0, 12.0], [7.0, 14.0]])
predicted_gpa = model.predict(new_data)
print(predicted_gpa)
```
上面的代码中,我们创建了模拟的样本数据,其中每个样本有两个特征(即学生的学时数和成绩),以及相应的GPA分数。然后,我们创建了一个`LinearRegression`对象,并使用`fit()`方法训练模型。接着,我们使用`predict()`方法对新数据进行预测,并输出结果。当然,您可以替换样本数据为您真实的数据,从而进行预测。
相关问题
用python通过线性回归方程编写预测考研概率的程序
Sure,我可以回答这个问题。首先,你需要准备考研成绩和其他相关因素的数据,并使用python中的scikit-learn库中的线性回归模型来拟合这些数据。具体实现过程如下:
1. 导入必要的库和数据
```python
import pandas as pd
from sklearn.linear_model import LinearRegression
# 读取考研成绩和相关因素的数据,例如GRE成绩,GPA,志愿者经历等
data = pd.read_csv('data.csv', header=0)
```
2. 将数据拆分为训练集和测试集,并对数据进行标准化处理
```python
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# 将数据拆分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(data.iloc[:, :-1], data.iloc[:, -1], test_size=0.2, random_state=1)
# 对数据进行标准化处理
scaler = StandardScaler().fit(X_train)
X_train_std = scaler.transform(X_train)
X_test_std = scaler.transform(X_test)
```
3. 使用线性回归模型进行拟合
```python
# 创建线性回归模型并拟合训练集数据
lr = LinearRegression()
lr.fit(X_train_std, y_train)
```
4. 使用模型进行预测
```python
# 对测试集数据进行预测
y_pred = lr.predict(X_test_std)
```
5. 计算模型的性能指标
```python
from sklearn.metrics import r2_score, mean_squared_error
print('R^2: %.2f' % r2_score(y_test, y_pred))
print('MSE: %.2f' % mean_squared_error(y_test, y_pred))
```
以上就是使用python通过线性回归方程编写预测考研概率的程序的大致步骤。
阅读全文
相关推荐
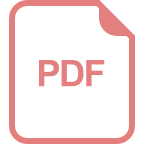
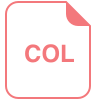
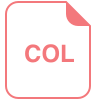
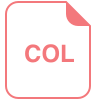
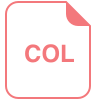
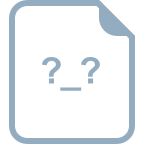
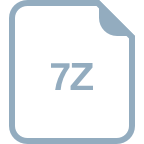
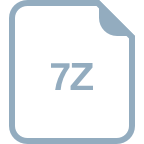
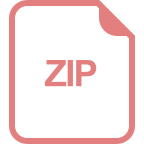
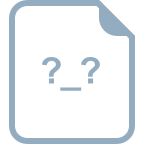
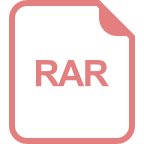
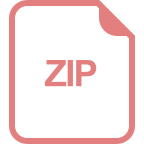