linux 串口通信 代码
时间: 2023-04-17 19:04:00 浏览: 247
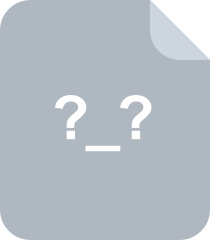
简单的Linux串口通信源代码

Linux下的串口通信代码可以使用C语言编写,主要包括以下步骤:
1. 打开串口设备文件,例如/dev/ttyS0或/dev/ttyUSB0。
2. 配置串口参数,包括波特率、数据位、停止位、校验位等。
3. 读取或写入串口数据,可以使用read()和write()函数。
4. 关闭串口设备文件。
下面是一个简单的Linux串口通信代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
// 打开串口设备文件
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 配置串口参数
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
// 读取或写入串口数据
char buf[256];
int n;
n = read(fd, buf, sizeof(buf));
if (n > 0) {
printf("Read %d bytes: %s\n", n, buf);
} else {
perror("read");
}
n = write(fd, "Hello, world!\n", 14);
if (n != 14) {
perror("write");
}
// 关闭串口设备文件
close(fd);
return 0;
}
```
这个代码示例打开了/dev/ttyS0串口设备文件,配置了波特率为9600,数据位为8,无校验位和停止位,然后读取了串口数据并输出,最后写入了一段字符串。注意,这个代码示例只是一个简单的示例,实际应用中需要根据具体需求进行修改和完善。
阅读全文
相关推荐

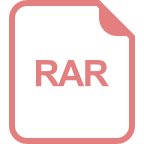
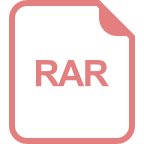
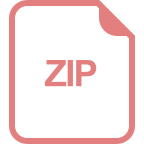
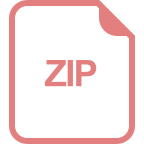
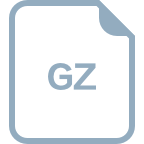
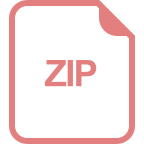
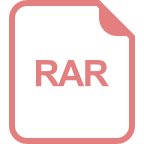
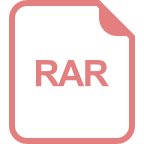
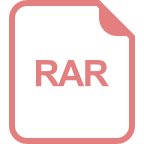
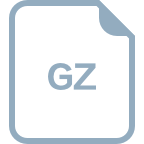
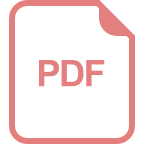
