基于JDBC的小型商品管理系统代码实现并在主方法中调用
时间: 2024-02-26 09:55:35 浏览: 69
好的,我会提供一个基于JDBC的小型商品管理系统的代码实现,并在主方法中调用。
我们假设有一个名为“products”的表,其中包含以下字段:id、name、price、quantity。下面是一个简单的商品管理系统的代码实现:
```
import java.sql.*;
public class ProductManagementSystem {
public static void main(String[] args) {
// 定义数据库连接信息
String url = "jdbc:mysql://localhost:3306/mydatabase?useSSL=false"; // 数据库连接地址
String user = "root"; // 数据库用户名
String password = "123456"; // 数据库密码
// 定义相关变量
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 加载JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 连接数据库
conn = DriverManager.getConnection(url, user, password);
// 创建Statement对象
stmt = conn.createStatement();
// 查询数据
String sql = "SELECT * FROM products";
rs = stmt.executeQuery(sql);
// 处理查询结果
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
double price = rs.getDouble("price");
int quantity = rs.getInt("quantity");
System.out.println("ID: " + id + ", Name: " + name + ", Price: " + price + ", Quantity: " + quantity);
}
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
// 释放资源
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在上面的代码中,我们首先定义了数据库连接信息,包括连接地址、用户名和密码。然后定义了三个变量,用于存储数据库连接、`Statement`对象和查询结果。接下来,我们通过`Class.forName()`方法来加载JDBC驱动程序,然后通过`DriverManager.getConnection()`方法来连接数据库。创建`Statement`对象后,我们可以使用`executeQuery()`方法来查询数据。最后,通过`while`循环来处理查询结果,并在控制台上打印输出。
在使用完JDBC后,我们通过`try-catch-finally`语句块来释放相关资源,包括`ResultSet`、`Statement`和`Connection`对象。
希望这个例子能够帮助您了解如何使用JDBC访问数据库,并实现一个简单的商品管理系统。
阅读全文
相关推荐


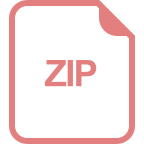
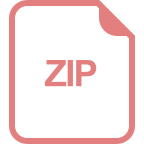
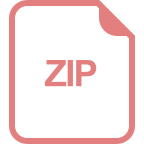
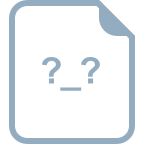
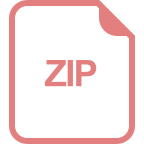
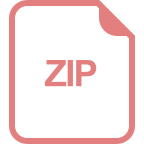
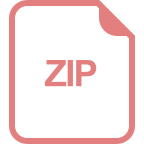
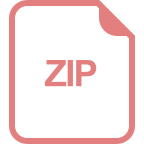
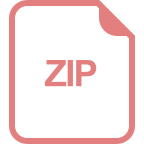
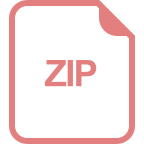
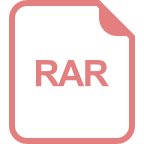
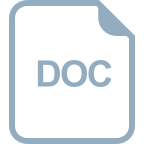
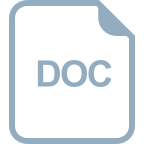
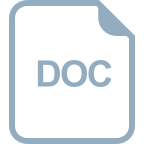