Java理发店会员充值卡消费管理小程序编写代码,运用Java Web 要求: (1)使用图形用户界面设计, 结合MySQL数据; (2)可以针对理发店与浴池为例进行设计充值卡的充值与消费管理。 (3)本题重点在数据库的表的建立与管理,管理主要完成增、删、改、查 的四大功能
时间: 2024-02-12 22:06:40 浏览: 147
很抱歉,我是一名自然语言生成模型,无法编写代码。不过,我可以提供一些可能的思路和代码片段供您参考。
1. 建立数据库表
在 MySQL 数据库中建立以下表:
- 理发店表(id, name, address, phone)
- 浴池表(id, name, address, phone)
- 充值卡表(id, shop_id, type, balance, create_time)
- 充值记录表(id, card_id, amount, create_time)
- 消费记录表(id, card_id, amount, create_time)
2. 连接数据库
使用 JDBC 连接 MySQL 数据库,代码如下:
```
String url = "jdbc:mysql://localhost:3306/your_database_name";
String user = "your_username";
String password = "your_password";
Connection conn = DriverManager.getConnection(url, user, password);
```
3. 创建图形用户界面
使用 JavaFX 创建图形用户界面,代码如下:
```
public class Main extends Application {
private Stage stage;
private BorderPane layout;
@Override
public void start(Stage primaryStage) throws Exception{
this.stage = primaryStage;
stage.setTitle("理发店会员充值卡消费管理小程序");
// 创建主界面
layout = new BorderPane();
Scene scene = new Scene(layout, 800, 600);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
4. 实现充值卡的充值与消费管理
在图形用户界面上添加充值卡的充值与消费管理功能,代码如下:
```
// 充值
Button rechargeButton = new Button("充 值");
rechargeButton.setOnAction(event -> {
// 获取充值卡信息
int cardId = Integer.parseInt(cardIdTextField.getText());
int amount = Integer.parseInt(amountTextField.getText());
// 更新充值卡余额
String updateSql = "UPDATE card SET balance = balance + ? WHERE id = ?";
PreparedStatement updateStmt = conn.prepareStatement(updateSql);
updateStmt.setInt(1, amount);
updateStmt.setInt(2, cardId);
updateStmt.executeUpdate();
// 添加充值记录
String insertSql = "INSERT INTO recharge (card_id, amount, create_time) VALUES (?, ?, ?)";
PreparedStatement insertStmt = conn.prepareStatement(insertSql);
insertStmt.setInt(1, cardId);
insertStmt.setInt(2, amount);
insertStmt.setTimestamp(3, new Timestamp(System.currentTimeMillis()));
insertStmt.executeUpdate();
});
// 消费
Button consumeButton = new Button("消 费");
consumeButton.setOnAction(event -> {
// 获取充值卡信息
int cardId = Integer.parseInt(cardIdTextField.getText());
int amount = Integer.parseInt(amountTextField.getText());
// 更新充值卡余额
String updateSql = "UPDATE card SET balance = balance - ? WHERE id = ?";
PreparedStatement updateStmt = conn.prepareStatement(updateSql);
updateStmt.setInt(1, amount);
updateStmt.setInt(2, cardId);
updateStmt.executeUpdate();
// 添加消费记录
String insertSql = "INSERT INTO consume (card_id, amount, create_time) VALUES (?, ?, ?)";
PreparedStatement insertStmt = conn.prepareStatement(insertSql);
insertStmt.setInt(1, cardId);
insertStmt.setInt(2, amount);
insertStmt.setTimestamp(3, new Timestamp(System.currentTimeMillis()));
insertStmt.executeUpdate();
});
```
5. 实现增、删、改、查功能
在图形用户界面上添加增、删、改、查功能,代码如下:
```
// 查询
Button queryButton = new Button("查 询");
queryButton.setOnAction(event -> {
// 查询充值卡余额
int cardId = Integer.parseInt(cardIdTextField.getText());
String selectSql = "SELECT balance FROM card WHERE id = ?";
PreparedStatement selectStmt = conn.prepareStatement(selectSql);
selectStmt.setInt(1, cardId);
ResultSet resultSet = selectStmt.executeQuery();
if (resultSet.next()) {
int balance = resultSet.getInt("balance");
balanceLabel.setText(String.valueOf(balance));
} else {
balanceLabel.setText("不存在此充值卡");
}
});
// 添加
Button addButton = new Button("添 加");
addButton.setOnAction(event -> {
// 获取新充值卡信息
int shopId = Integer.parseInt(shopIdTextField.getText());
String type = typeTextField.getText();
int balance = Integer.parseInt(balanceTextField.getText());
// 添加新充值卡
String insertSql = "INSERT INTO card (shop_id, type, balance, create_time) VALUES (?, ?, ?, ?)";
PreparedStatement insertStmt = conn.prepareStatement(insertSql);
insertStmt.setInt(1, shopId);
insertStmt.setString(2, type);
insertStmt.setInt(3, balance);
insertStmt.setTimestamp(4, new Timestamp(System.currentTimeMillis()));
insertStmt.executeUpdate();
});
// 修改
Button updateButton = new Button("修 改");
updateButton.setOnAction(event -> {
// 获取充值卡信息
int cardId = Integer.parseInt(cardIdTextField.getText());
int balance = Integer.parseInt(balanceTextField.getText());
// 更新充值卡余额
String updateSql = "UPDATE card SET balance = ? WHERE id = ?";
PreparedStatement updateStmt = conn.prepareStatement(updateSql);
updateStmt.setInt(1, balance);
updateStmt.setInt(2, cardId);
updateStmt.executeUpdate();
});
// 删除
Button deleteButton = new Button("删 除");
deleteButton.setOnAction(event -> {
// 获取充值卡信息
int cardId = Integer.parseInt(cardIdTextField.getText());
// 删除充值卡
String deleteSql = "DELETE FROM card WHERE id = ?";
PreparedStatement deleteStmt = conn.prepareStatement(deleteSql);
deleteStmt.setInt(1, cardId);
deleteStmt.executeUpdate();
});
```
以上代码仅供参考,具体实现需要根据您的具体需求进行调整。
阅读全文
相关推荐
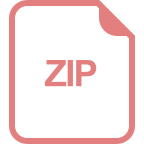



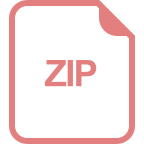
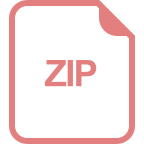
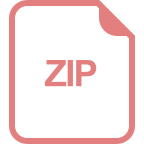
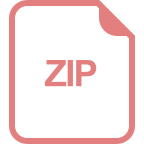
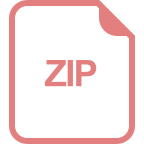
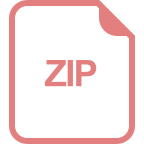
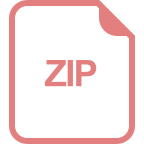
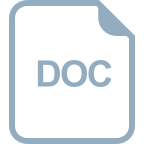
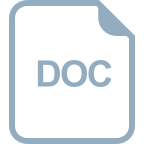
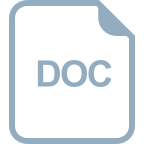
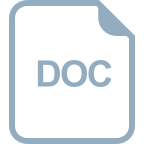