python压缩字符串
时间: 2024-11-12 20:16:39 浏览: 5
在Python中,有多种方法可以压缩字符串,主要取决于你的需求,是否需要保持可读性或者只是减小存储空间。以下是几种常见的字符串压缩技术:
1. **gzip 和 zlib**:Python标准库提供`gzip`和`zlib`模块,可以用于对数据进行高效的压缩。例如,你可以使用`gzip.compress()`和`gzip.decompress()`函数来进行操作。
```python
import gzip
data = "your string here"
compressed_data = gzip.compress(data.encode())
# 使用gzip.decompress()解压
```
2. **Base64编码**:这是一种简单的文本数据压缩方式,将原始字符串转换成ASCII字符集表示。虽然这不是真正的压缩,但可以减少二进制数据的大小。
```python
import base64
compressed_data = base64.b64encode(data.encode()).decode()
```
3. **LZW 或 Huffman 编码**:对于特定类型的字符串,比如文本文件,可以使用更专业的压缩算法,如LZW或Huffman编码。不过这通常需要自定义实现或者使用第三方库,如`lzma`(也是zlib的一部分)或`pyzopfli`等。
```python
import lzma
compressed_data = lzma.compress(data.encode())
```
相关问题
python 压缩字符串长度
根据提供的引用内容,以下是一个解决方案来压缩字符串长度的Python代码示例:
```python
def compress(chars):
if len(chars) == 0:
return 0
if len(chars) == 1:
return 1
write = 0
count = 1
for read in range(1, len(chars)):
if chars[read] == chars[read - 1]:
count += 1
else:
chars[write] = chars[read - 1]
write += 1
if count > 1:
for digit in str(count):
chars[write] = digit
write += 1
count = 1
chars[write] = chars[-1]
write += 1
if count > 1:
for digit in str(count):
chars[write] = digit
write += 1
return write
chars = ["a", "a", "b", "b", "c", "c", "c"]
compressed_length = compress(chars)
print("Compressed length:", compressed_length)
print("Compressed chars:", chars[:compressed_length])
```
这段代码使用了两个指针,`read`和`write`,以及一个计数器`count`来实现原地修改数组并压缩字符串长度。它遍历输入数组,如果当前字符与前一个字符相同,则增加计数器`count`的值;否则,将前一个字符和计数器的值写入数组中,并更新`write`指针。最后,返回`write`指针的值作为压缩后的字符串长度。
python解压缩字符串
要在Python中解压缩字符串,可以使用gzip模块中的gzip_decompress函数。这个函数接受一个被压缩的字符串作为参数,并返回解压缩后的字符串。下面是一个使用gzip_decompress函数解压缩字符串的示例代码:
```python
import gzip
def gzip_decompress(buf):
with gzip.GzipFile(fileobj=buf) as f:
result = f.read()
return result
compressed_string = "压缩后的字符串"
decompressed_string = gzip_decompress(compressed_string)
print(decompressed_string)
```
请注意,这个示例代码中的compressed_string是一个被压缩的字符串,你需要将其替换为你要解压缩的实际字符串。
#### 引用[.reference_title]
- *1* *3* [PTA 1078 字符串压缩与解压(Python3)](https://blog.csdn.net/weixin_55730361/article/details/126328055)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [python gzip 压缩/解压缩 字符串](https://blog.csdn.net/qq_43645782/article/details/113356724)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
阅读全文
相关推荐
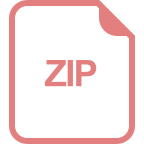
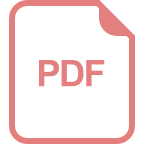
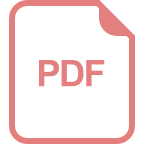




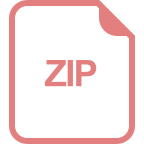
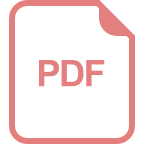
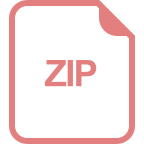
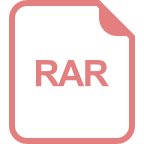
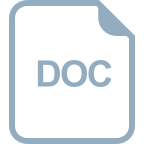
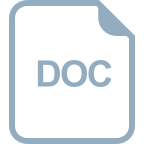
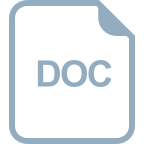
