用C语言将串口接收到的字符串以十六进制的形式发布ROStopic数据
时间: 2024-02-09 21:10:45 浏览: 74
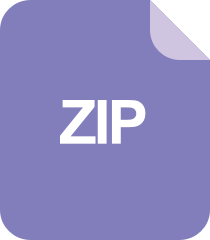
Linux C语言实现串口收发数据

好的,这个问题需要涉及到串口通信、ROS和十六进制转换等知识。我将分步骤回答你的问题:
1. 串口通信
在C语言中,串口通信需要使用串口通信库,比如常用的有termios.h、fcntl.h和unistd.h等库。通过这些库,我们可以打开串口、设置串口参数、读取串口数据等。下面是一个简单的串口通信代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
exit(1);
}
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
tcsetattr(fd, TCSANOW, &options);
char buf[256];
int n;
while (1) {
n = read(fd, buf, 256);
if (n > 0) {
buf[n] = '\0';
printf("Received: %s\n", buf);
}
}
close(fd);
return 0;
}
```
这段代码打开了/dev/ttyUSB0设备,设置了波特率为9600,并且循环读取串口数据并打印出来。
2. ROS
ROS是机器人操作系统,是一个开源的机器人软件框架。在ROS中,我们可以通过发布和订阅话题来实现不同节点之间的通信。下面是一个简单的ROS节点示例:
```c++
#include "ros/ros.h"
#include "std_msgs/String.h"
int main(int argc, char **argv)
{
ros::init(argc, argv, "talker");
ros::NodeHandle n;
ros::Publisher chatter_pub = n.advertise<std_msgs::String>("chatter", 1000);
ros::Rate loop_rate(10);
int count = 0;
while (ros::ok())
{
std_msgs::String msg;
std::stringstream ss;
ss << "hello world " << count;
msg.data = ss.str();
ROS_INFO("%s", msg.data.c_str());
chatter_pub.publish(msg);
ros::spinOnce();
loop_rate.sleep();
++count;
}
return 0;
}
```
这个ROS节点会发布一个名为chatter的话题,并且循环发布字符串"hello world "和一个计数器的值。
3. 十六进制转换
在C语言中,我们可以使用sprintf函数将一个整数转换成十六进制字符串。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num = 255;
char hex_str[3];
sprintf(hex_str, "%02X", num);
printf("Hex string: %s\n", hex_str);
return 0;
}
```
这个代码将整数255转换成十六进制字符串"FF"。
综合以上三个步骤,我们可以编写一个将串口接收到的字符串以十六进制的形式发布ROS话题的程序。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include "ros/ros.h"
#include "std_msgs/String.h"
int main(int argc, char **argv)
{
ros::init(argc, argv, "serial_node");
ros::NodeHandle n;
ros::Publisher serial_pub = n.advertise<std_msgs::String>("serial_data", 1000);
ros::Rate loop_rate(10);
int fd;
struct termios options;
fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
exit(1);
}
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
tcsetattr(fd, TCSANOW, &options);
char buf[256];
int n;
while (ros::ok()) {
n = read(fd, buf, 256);
if (n > 0) {
std_msgs::String msg;
char hex_str[3];
for (int i = 0; i < n; i++) {
sprintf(hex_str, "%02X", (unsigned char)buf[i]);
msg.data += hex_str;
}
serial_pub.publish(msg);
ROS_INFO("Received: %s", msg.data.c_str());
}
ros::spinOnce();
loop_rate.sleep();
}
close(fd);
return 0;
}
```
这个程序会循环读取串口数据,并将每个字节转换成两个十六进制字符,并将它们连接成一个字符串,最后发布ROS话题。注意,在ROS中节点需要不断调用ros::spinOnce()函数来处理消息。
阅读全文
相关推荐
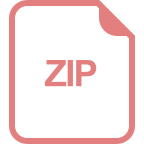


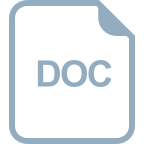









