js怎么判断数组中是否有某个元素
时间: 2023-07-08 15:47:31 浏览: 59
在JavaScript中,可以使用`Array.includes()`方法来判断一个数组中是否包含某个元素。`Array.includes()`方法返回一个布尔值,表示数组中是否包含指定的元素。
例如,假设有一个数组`arr`,我们想要判断其中是否包含数字`3`,可以使用以下代码:
```
const arr = [1, 2, 3, 4, 5];
if (arr.includes(3)) {
console.log('数组中包含数字3');
} else {
console.log('数组中不包含数字3');
}
```
如果数组`arr`中包含数字`3`,则会输出`数组中包含数字3`,否则会输出`数组中不包含数字3`。
需要注意的是,`Array.includes()`方法在判断字符串和数字时是严格匹配的,即必须是同一种类型的数据才能匹配成功。如果要判断一个对象或数组是否在数组中,可以使用`Array.indexOf()`方法或者`Array.find()`方法来判断。
相关问题
js中判断一个数组中是否有某个元素
在 JavaScript 中,你可以使用 `Array.includes()` 方法来判断一个数组中是否包含某个元素。个方法返回一个布尔值,表示该元素是否存在于数组中。
以下是使用 `Array.includes()` 方法来判断一个数组中是否包含某个元素的示例代码:
```javascript
const array = [1, 2, 3, 4, 5];
const element = 3;
if (array.includes(element)) {
console.log("数组中包含该元素");
} else {
console.log("数组中不包含该元素");
}
```
在上面的示例代码中,我们定义了一个数组 `array`,并判断其中是否包含元素 `3`。如果数组中包含该元素,则输出 "数组中包含该元素";否则输出 "数组中不包含该元素"。
js判断数组中是否有某个值
你可以使用JavaScript中的`includes()`方法来判断数组中是否包含某个特定的值。这个方法会返回一个布尔值来表示是否找到了指定的值。
下面是一个示例代码:
```javascript
let array = [1, 2, 3, 4, 5];
let value = 3;
if (array.includes(value)) {
console.log("数组中包含指定的值");
} else {
console.log("数组中不包含指定的值");
}
```
在上面的例子中,数组`array`包含数字1到5,我们使用`includes()`方法来判断数组中是否包含值为3的元素。如果找到了,则输出"数组中包含指定的值",否则输出"数组中不包含指定的值"。
你也可以使用`indexOf()`方法来判断数组中是否包含某个值。如果值存在于数组中,则`indexOf()`方法会返回值的索引,否则返回-1。你可以利用这个特性来判断是否找到了指定的值。
```javascript
let array = [1, 2, 3, 4, 5];
let value = 3;
if (array.indexOf(value) !== -1) {
console.log("数组中包含指定的值");
} else {
console.log("数组中不包含指定的值");
}
```
这两种方法都可以用来判断数组中是否包含某个值,你可以根据自己的需求选择使用。
相关推荐
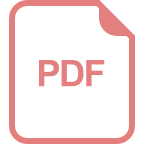
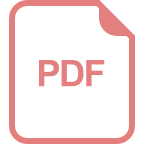












