java贪心算法背包问题
时间: 2023-11-10 09:04:20 浏览: 101
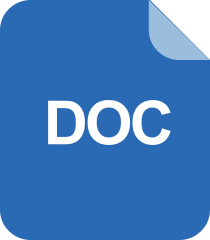
贪心算法 背包问题
背包问题是一个经典的动态规划问题,但也可以使用贪心算法来解决。具体来说,可以按照物品的单位重量价值进行排序,然后依次将单位重量价值最高的物品放入背包中,直到背包无法再放入为止。
Java代码实现如下:
```java
public class KnapsackProblem {
public static void main(String[] args) {
int[] weights = {2, 3, 4, 5};
int[] values = {3, 4, 5, 6};
int capacity = 8;
double maxValue = knapsack(weights, values, capacity);
System.out.println("Max value: " + maxValue);
}
public static double knapsack(int[] weights, int[] values, int capacity) {
int n = weights.length;
double[] unitValues = new double[n];
for (int i = 0; i < n; i++) {
unitValues[i] = (double) values[i] / weights[i];
}
// 按照单位重量价值排序
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (unitValues[i] < unitValues[j]) {
swap(unitValues, i, j);
swap(weights, i, j);
swap(values, i, j);
}
}
}
// 贪心选择物品
double maxValue = 0;
for (int i = 0; i < n && capacity > 0; i++) {
if (weights[i] <= capacity) {
maxValue += values[i];
capacity -= weights[i];
} else {
maxValue += unitValues[i] * capacity;
capacity = 0;
}
}
return maxValue;
}
private static void swap(double[] arr, int i, int j) {
double temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
```
阅读全文
相关推荐




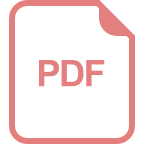
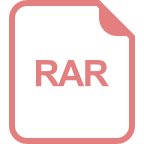







