from ecloud import CMSSEcloudOcrClient import json import os from collections import OrderedDict accesskey = '357c19f09220408cb3650e8ca8a418c7' secretkey = 'f5f4f10272284ec3829460e0a65f5cd2' url = 'https://api-wuxi-1.cmecloud.cn:8443' def request_webimage(imagepath): requesturl = '/api/ocr/v1/webimage' try: ocr_client = CMSSEcloudOcrClient(accesskey, secretkey, url) response = ocr_client.request_ocr_service_file(requestpath=requesturl, imagepath=imagepath) json_data = json.loads(response.text, object_pairs_hook=OrderedDict) # 使用OrderedDict保证输出的文本有序 print(json.dumps(json_data, indent=4, ensure_ascii=False)) # 使用json.dumps()方法美化输出 # 将数据保存到txt文件 with open('output.txt', 'a', encoding='utf-8') as f: f.write(json.dumps(json_data, indent=4, ensure_ascii=False)) f.write('\n') # 每个json数据之间换行分隔 except ValueError as e: print(e) if __name__ == "__main__": folder_path = "C:\\Users\\Administrator\\Desktop\\pics" # 修改为实际的文件夹路径 for filename in os.listdir(folder_path): if filename.endswith(".jpg") or filename.endswith(".jpeg") or filename.endswith(".png"): imagepath = os.path.join(folder_path, filename) request_webimage(imagepath)帮我改一下这个代码,使用NER标记输出的json文件中的数据
时间: 2024-02-29 12:56:48 浏览: 22
好的,我可以帮你修改这段代码,让它能够使用NER标记输出的JSON文件中的数据。你需要使用一个NER模型来对文本进行标记,可以使用开源的NER模型如SpaCy或Stanford NER。
假设你已经安装了SpaCy,可以按照以下步骤修改代码:
1. 在代码开头导入SpaCy库:`import spacy`
2. 加载SpaCy的NER模型:`nlp = spacy.load("en_core_web_sm")`,这里使用的是英文模型,你可以根据需要选择其他语言的模型。
3. 在`request_webimage`函数中,将返回的JSON数据传递给SpaCy的`nlp`对象进行处理:`doc = nlp(json_data["text"])`
4. 遍历文档中的实体,将它们存储到一个列表中:`entities = []`,然后使用`doc.ents`属性获取所有的实体,并将它们存储到列表中:`entities.extend(doc.ents)`
5. 将实体列表添加到JSON数据中:`json_data["entities"] = entities`
最终修改后的代码如下:
```
from ecloud import CMSSEcloudOcrClient
import json
import os
from collections import OrderedDict
import spacy
accesskey = '357c19f09220408cb3650e8ca8a418c7'
secretkey = 'f5f4f10272284ec3829460e0a65f5cd2'
url = 'https://api-wuxi-1.cmecloud.cn:8443'
nlp = spacy.load("en_core_web_sm")
def request_webimage(imagepath):
requesturl = '/api/ocr/v1/webimage'
try:
ocr_client = CMSSEcloudOcrClient(accesskey, secretkey, url)
response = ocr_client.request_ocr_service_file(requestpath=requesturl, imagepath=imagepath)
json_data = json.loads(response.text, object_pairs_hook=OrderedDict)
doc = nlp(json_data["text"])
entities = []
for ent in doc.ents:
entities.append({
"text": ent.text,
"start_char": ent.start_char,
"end_char": ent.end_char,
"label": ent.label_
})
json_data["entities"] = entities
# 使用OrderedDict保证输出的文本有序
print(json.dumps(json_data, indent=4, ensure_ascii=False))
# 将数据保存到txt文件
with open('output.txt', 'a', encoding='utf-8') as f:
f.write(json.dumps(json_data, indent=4, ensure_ascii=False))
f.write('\n') # 每个json数据之间换行分隔
except ValueError as e:
print(e)
if __name__ == "__main__":
folder_path = "C:\\Users\\Administrator\\Desktop\\pics" # 修改为实际的文件夹路径
for filename in os.listdir(folder_path):
if filename.endswith(".jpg") or filename.endswith(".jpeg") or filename.endswith(".png"):
imagepath = os.path.join(folder_path, filename)
request_webimage(imagepath)
```
这样修改后,输出的JSON数据中将包含一个名为"entities"的字段,其中存储了所有的实体及其标签。你可以根据需要修改实体的格式和标签。
相关推荐








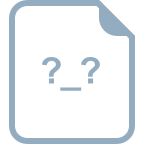
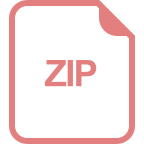
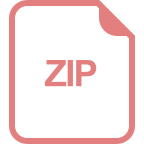