from PyQt5 import QtCore, QtGui, QtWidgets from show1 import Ui_Form1 from show2 import Ui_Form2 from show3 import Ui_Form3 class Ui_Form(object): def setupUi(self, Form): Form.setObjectName("Form") Form.resize(400, 300) self.pushButton = QtWidgets.QPushButton(Form) self.pushButton.setGeometry(QtCore.QRect(90, 60, 191, 51)) font = QtGui.QFont() font.setPointSize(9) self.pushButton.setFont(font) self.pushButton.setObjectName("pushButton") self.pushButton_2 = QtWidgets.QPushButton(Form) self.pushButton_2.setGeometry(QtCore.QRect(90, 110, 191, 51)) self.pushButton_2.setObjectName("pushButton_2") self.pushButton_3 = QtWidgets.QPushButton(Form) self.pushButton_3.setGeometry(QtCore.QRect(90, 160, 191, 51)) self.pushButton_3.setObjectName("pushButton_3") self.retranslateUi(Form) QtCore.QMetaObject.connectSlotsByName(Form) def retranslateUi(self, Form): _translate = QtCore.QCoreApplication.translate Form.setWindowTitle(_translate("Form", "Form")) self.pushButton.setText(_translate("Form", "无人机群显示")) self.pushButton_2.setText(_translate("Form", "无人机群数据分析展示")) self.pushButton_3.setText(_translate("Form", "无人机群飞行轨迹展示")) class MainWindow(QtWidgets.QMainWindow): def __init__(self): super().__init__() self.ui = Ui_Form() self.ui.setupUi(self) self.ui.pushButton.clicked.connect(self.showForm1) self.ui.pushButton_2.clicked.connect(self.showForm2) self.ui.pushButton_3.clicked.connect(self.showForm3) def showForm1(self): self.form1 = QtWidgets.QWidget() self.ui1 = Ui_Form1() self.ui1.setupUi(self.form1) self.form1.show() def showForm2(self): self.form2 = QtWidgets.QWidget() self.ui2 = Ui_Form2() self.ui2.setupUi(self.form2) self.form2.show() def showForm3(self): self.form3 = QtWidgets.QWidget() self.ui3 = Ui
时间: 2024-03-14 19:49:21 浏览: 114
_Form3() self.ui3.setupUi(self.form3) self.form3.show()
这段代码是一个基于PyQt5的GUI程序,它创建了一个主窗口并在窗口中添加了三个按钮。当用户单击按钮时,程序会打开另外三个窗口,分别用于显示无人机群的位置、数据分析和飞行轨迹。这个程序的核心是MainWindow类,它继承自QtWidgets.QMainWindow,并在__init__方法中创建了UI对象并连接了三个按钮的clicked信号到对应的槽函数showForm1、showForm2和showForm3。这三个槽函数分别创建了对应的窗口对象,并在窗口中添加了对应的UI组件,并将窗口显示出来。
相关问题
from PyQt5 import QtCore, QtGui, QtWidgets
这段代码是用于导入 PyQt5 模块中的 QtCore、QtGui 和 QtWidgets 模块。这些模块是用于创建基于 Qt 框架的 GUI 应用程序的核心模块。QtCore 模块包含了 Qt 的核心非 GUI 功能,如信号与槽机制、事件处理等。QtGui 模块包含了 Qt 的 GUI 类和方法,如窗口、按钮、标签等。QtWidgets 模块包含了 Qt 的现代化的用户界面控件,如滑块、输入框、列表控件等。导入这些模块后,可以使用其中的类和方法来构建 GUI 应用程序。
解释代码 from PyQt5 import QtCore,QtGui,QtWidgets
这段代码是Python中使用PyQt5模块导入QtCore、QtGui和QtWidgets模块的语句。这三个模块是PyQt5中最常用的模块,提供了实现图形界面和交互式应用程序所需的类和函数。QtCore提供了保持数据的数据结构,QtGui包含了窗口系统的基本组件(如标签、文本框、按钮等等)以及绘图和事件处理等功能,而QtWidgets则提供了用于创建窗口、对话框、菜单、工具条等UI元素的类。
阅读全文
相关推荐
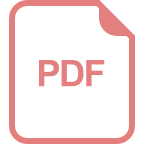
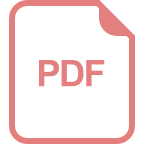
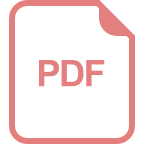












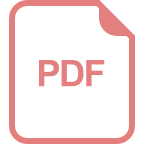
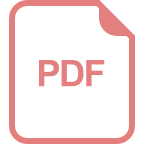