编写程序从键盘输入三个数输出三个数最大值
时间: 2023-04-11 20:01:01 浏览: 148
这个问题可以回答。以下是一个示例程序:
```
#include <stdio.h>
int main() {
int a, b, c, max;
printf("请输入三个数:");
scanf("%d %d %d", &a, &b, &c);
max = a;
if (b > max) {
max = b;
}
if (c > max) {
max = c;
}
printf("最大值是:%d\n", max);
return 0;
}
```
相关问题
汇编程序实现:从键盘输入三个数输出最大值
在汇编语言中,实现从键盘输入三个数并输出其中的最大值,通常需要几个步骤。这里以x86架构为例,使用MASM(Microsoft Assembler)编写简单示例:
```assembly
; 使用in指令从键盘读取数据
section .data
format db '请输入第一个数:$'
prompt db '请输入第二个数:$'
prompt3 db '请输入第三个数:$'
maxmsg db '最大值是:$'
section .bss
num1 resd 1
num2 resd 1
num3 resd 1
max resd 1
section .text
global _start
_start:
; 提示用户输入,并保存到内存
mov eax, 4 ; sys_write syscall number
mov ebx, 1 ; stdout file descriptor
mov ecx, prompt
mov edx, len1 ; length of the prompt
int 0x80 ; call kernel
push num1 ; store address of num1 on stack
push eax ; save return value from read syscall
call getchar ; read first number
add esp, 8 ; clean up stack
mov eax, 4 ; sys_read syscall number
mov ebx, 0 ; stdin file descriptor
mov ecx, [num1] ; load the address of num1 into ecx
mov edx, 4 ; assuming a 32-bit integer
int 0x80 ; call kernel
; 同样的过程对第二个和第三个数进行
mov ecx, prompt3
mov edx, len3 ; length of the prompt
int 0x80 ; write prompt for second number
push num2
call getchar
add esp, 8
mov ecx, maxmsg
mov edx, lenmax ; length of "max is:"
int 0x80 ; write "max is:"
push num3
call getchar
add esp, 8
; 比较三个数并找到最大值
mov eax, [num1]
cmp eax, [num2]
jg max_found1
xchg eax, [num2]
max_found1:
cmp eax, [num3]
jg max_found2
max_found2:
; 最大值已经存在num1中,现在输出它
mov ebx, 1 ; sys_write syscall number
mov ecx, maxmsg
mov edx, lenmax ; length of "max is:"
int 0x80 ; write "max is:"
mov ebx, [num1] ; convert to string and output
mov ecx, str_max
mov edx, 4
int 0x80
exit_program:
mov eax, 1 ; sys_exit syscall number
xor ebx, ebx ; exit code 0
int 0x80 ; call kernel
len1 equ $ - prompt
len2 equ $ - maxmsg
str_max db ' %d', 10 ; format specifier for an integer
getchar:
; 这里省略实际的getchar函数实现,因为它会依赖于平台
; 返回值存放在eax,然后清除堆栈
ret
```
这个示例仅作教学演示,实际的`getchar`函数实现取决于操作系统和平台,通常需要使用中断或系统调用来从键盘获取字符。
编写一个c++程序 要求是从键盘中依次输入三个数,计算并输出三个数的最大值,用三项条件运算符
好的,下面是代码:
```c++
#include <iostream>
using namespace std;
int main() {
int a, b, c;
cout << "请输入三个数:";
cin >> a >> b >> c;
int maxNum = (a > b) ? ((a > c) ? a : c) : ((b > c) ? b : c);
cout << "最大值为:" << maxNum << endl;
return 0;
}
```
程序会先让用户依次输入三个数,然后利用三项条件运算符比较三个数的大小,找出最大值并输出。
阅读全文
相关推荐
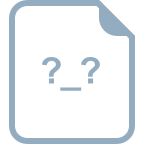
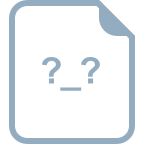













