哈里斯鹰优化算法的python
时间: 2024-12-12 18:15:20 浏览: 5
哈里斯鹰优化算法(Harris Hawks Optimization, HHO)是一种基于自然界中哈里斯鹰捕猎行为的元启发式优化算法。该算法通过模拟哈里斯鹰的协作捕猎策略来寻找问题的最优解。以下是哈里斯鹰优化算法的Python实现示例:
```python
import numpy as np
class HHO:
def __init__(self, objective_function, bounds, n_hawks, max_iter):
self.objective_function = objective_function
self.bounds = bounds
self.n_hawks = n_hawks
self.max_iter = max_iter
self.dim = len(bounds)
self.population = np.random.uniform(low=[b[0] for b in bounds],
high=[b[1] for b in bounds],
size=(self.n_hawks, self.dim))
self.fitness = np.array([self.objective_function(individual) for individual in self.population])
self.best_index = np.argmin(self.fitness)
self.best_solution = self.population[self.best_index]
self.best_fitness = self.fitness[self.best_index]
def optimize(self):
for iteration in range(self.max_iter):
for i in range(self.n_hawks):
E1 = 2 * (1 - (iteration / self.max_iter))
E0 = -1 + np.random.random() * 2
J = 2 * (1 - np.random.random())
if np.abs(E0) >= 1:
# Exploration on the population
q = np.random.random()
rand_hawk_index = np.random.randint(0, self.n_hawks)
X_rand = self.population[rand_hawk_index]
if q < 0.5:
self.population[i] = X_rand - np.random.random() * np.abs(X_rand - 2 * np.random.random() * self.population[i])
else:
self.population[i] = (self.best_solution - self.population.mean(0)) - np.random.random() * (self.bounds[:, 0] + np.random.random() * (self.bounds[:, 1] - self.bounds[:, 0]))
else:
# Exploitation by team work
if np.random.random() >= 0.5:
Y = self.best_solution - E1 * np.abs(self.best_solution - 2 * np.random.random() * self.population[i])
else:
X_rand = self.population[np.random.randint(0, self.n_hawks)]
Y = X_rand - E1 * np.abs(X_rand - 2 * np.random.random() * self.population[i])
self.population[i] = Y
self.fitness = np.array([self.objective_function(individual) for individual in self.population])
current_best_index = np.argmin(self.fitness)
if self.fitness[current_best_index] < self.best_fitness:
self.best_solution = self.population[current_best_index]
self.best_fitness = self.fitness[current_best_index]
print(f"Iteration {iteration+1}, Best Fitness: {self.best_fitness}")
return self.best_solution, self.best_fitness
# Example usage
if __name__ == "__main__":
def objective_function(x):
return np.sum(x**2)
bounds = [(-10, 10), (-10, 10)]
n_hawks = 10
max_iter = 50
hho = HHO(objective_function, bounds, n_hawks, max_iter)
best_solution, best_fitness = hho.optimize()
print(f"Best Solution: {best_solution}, Best Fitness: {best_fitness}")
```
阅读全文
相关推荐


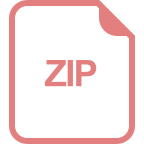
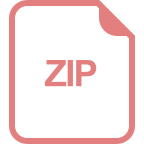


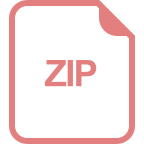
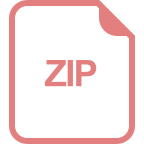
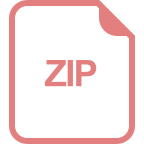
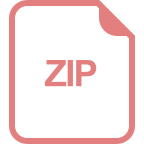
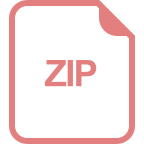
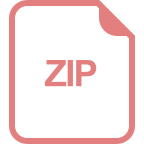

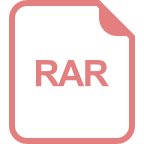
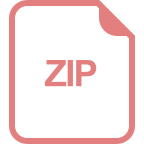
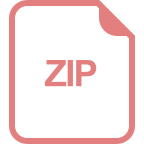

