python编程实现十进制到度分秒的转换
时间: 2024-09-27 07:10:55 浏览: 46
在Python中,你可以创建一个函数来将十进制的小数值转换为度、分、秒的形式。这里是一个简单的例子:
```python
def decimal_to_degrees_minutes_seconds(decimal):
# 确保输入值在0到60之间,超过这个范围的值会被舍去
decimal %= 60
seconds = int(decimal)
minutes = int((decimal - seconds) * 60)
degrees = int((decimal - seconds - minutes / 60) * 3600)
return f"{degrees}° {minutes}\' {seconds}\""
# 测试函数
print(decimal_to_degrees_minutes_seconds(365.8)) # 输出: "1° 35\' 48\""
```
这个函数首先取余数得到小数部分,然后依次计算出秒、分钟和度,并以字符串形式返回结果。注意这里假设了小数部分始终小于等于1小时(即60分钟),实际应用中可能需要添加更复杂的错误检查。
相关问题
python编程实现十进制到度分秒的转换,并且该编程能 ① 判断用户输入的数据是否能够转换成浮点数; ② 判断用户输入的数据是否在0~360°范围之内; ③ 使用f-string格式化字符串语句格式化转换后弧度的输出格式。
在Python中,你可以创建一个函数来实现十进制到度分秒的转换,并同时满足上述三个条件。这里是一个简单的示例:
```python
def decimal_to_degrees_minutes_seconds(decimal):
try:
# 判断输入能否转换为浮点数
if not isinstance(decimal, (int, float)):
raise ValueError("输入数据必须是数字")
# 转换为浮点数并判断范围
if decimal < 0 or decimal > 360:
raise ValueError("输入值应在0到360度之间")
# 将弧度转换为度、分、秒
degrees = round(decimal)
minutes, seconds = divmod((decimal - degrees) * 60, 60)
# 格式化并返回结果
result = f"{degrees}°{minutes:02d}'{seconds:02d}\""
except ValueError as ve:
print(ve)
return None
return result
# 用户输入
input_decimal = input("请输入一个0到360之间的角度(十进制形式):")
result = decimal_to_degrees_minutes_seconds(float(input_decimal))
if result is not None:
print(result)
编程实现十进制度到度分秒的转换
将十进制数转换为度、分、秒的格式通常涉及到整除和取余的过程。下面是一个简单的Python示例,用于将十进制时间(假设是最小单位秒)转换为度分秒:
```python
def decimal_to_degrees_minutes_seconds(decimal_time):
seconds = decimal_time % 60 # 取余得到剩余秒数
minutes = (decimal_time // 60) % 60 # 整除60并取余得到分钟数
degrees = (decimal_time // (60 * 60)) % 60 # 再次整除(60*60)得到小时数,然后取余得到度数
return degrees, minutes, seconds
# 示例
decimal_time = 157894
degrees, minutes, seconds = decimal_to_degrees_minutes_seconds(decimal_time)
print(f"{degrees}° {minutes}′ {seconds}\"")
阅读全文
相关推荐






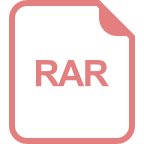








