vue实现半圆形运动轨迹
时间: 2023-09-06 08:11:57 浏览: 123
要实现半圆形运动轨迹,可以使用 Vue.js 和 SVG。
首先,创建一个 SVG 元素,设置它的宽度和高度,并添加一个圆形元素:
```html
<svg width="200" height="200">
<circle cx="100" cy="100" r="10" fill="red" />
</svg>
```
然后,在 Vue 组件中,可以使用 `setInterval` 函数来定时更新圆形的位置,从而实现半圆形运动轨迹。具体来说,可以计算出圆形的 x 和 y 坐标,然后使用 `setAttribute` 方法来更新圆形元素的 `cx` 和 `cy` 属性。
```html
<template>
<div>
<svg ref="svg" width="200" height="200">
<circle ref="circle" r="10" fill="red" />
</svg>
</div>
</template>
<script>
export default {
mounted() {
const svg = this.$refs.svg;
const circle = this.$refs.circle;
let angle = 0;
setInterval(() => {
const x = 100 + Math.cos(angle) * 90;
const y = 100 + Math.sin(angle) * 90;
circle.setAttribute('cx', x);
circle.setAttribute('cy', y);
angle += 0.1;
}, 50);
},
};
</script>
```
在上面的代码中,`angle` 变量表示圆形当前所在的角度,每次更新时增加 0.1,从而让圆形沿着半圆形运动。`x` 和 `y` 变量分别表示圆形的 x 和 y 坐标,计算公式如下:
```
x = cx + r * cos(angle)
y = cy + r * sin(angle)
```
其中,`cx` 和 `cy` 是半圆形的中心坐标,`r` 是圆形的半径。在本例中,`cx` 和 `cy` 分别为 100,`r` 为 90,这样就可以得到圆形在半圆形上运动的轨迹了。
阅读全文
相关推荐
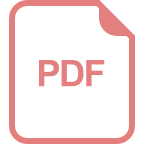
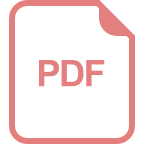
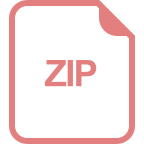
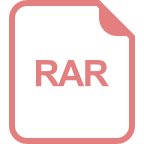
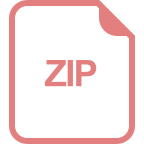
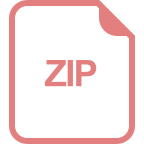
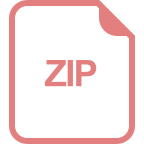
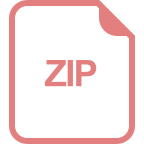
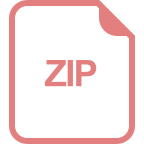
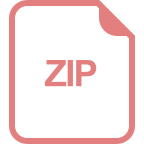
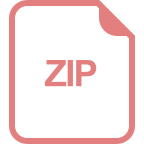
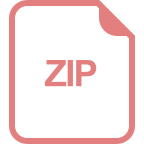
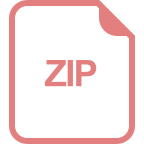