用pygame设计一款简单游戏,有开始游戏gui界面。要求很多小球下落是用挡板接住,每接到一次加一分,没接到减两分,累计达到10分取得胜利,结束游戏。
时间: 2023-06-17 15:02:30 浏览: 37
好的,下面是一个简单的游戏设计:
1. 导入 pygame 模块和 sys 模块
```python
import pygame
import sys
```
2. 初始化 pygame 和游戏界面
```python
pygame.init()
# 设置游戏界面大小
size = width, height = 600, 400
screen = pygame.display.set_mode(size)
# 设置游戏界面标题
pygame.display.set_caption("小球接球游戏")
```
3. 定义小球类和挡板类
```python
class Ball:
def __init__(self, x, y, radius, speed):
self.x = x
self.y = y
self.radius = radius
self.speed = speed
self.color = (255, 0, 0)
def move(self):
self.y += self.speed
def draw(self):
pygame.draw.circle(screen, self.color, (self.x, self.y), self.radius)
class Paddle:
def __init__(self, x, y, width, height, speed):
self.x = x
self.y = y
self.width = width
self.height = height
self.speed = speed
self.color = (0, 255, 0)
def move_left(self):
self.x -= self.speed
def move_right(self):
self.x += self.speed
def draw(self):
pygame.draw.rect(screen, self.color, (self.x, self.y, self.width, self.height))
```
4. 定义游戏开始界面和游戏结束界面
```python
def start_screen():
font = pygame.font.Font(None, 36)
text = font.render("点击空格键开始游戏", True, (255, 255, 255))
text_rect = text.get_rect()
text_rect.centerx = screen.get_rect().centerx
text_rect.centery = screen.get_rect().centery
screen.blit(text, text_rect)
pygame.display.update()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
return
def end_screen(score):
font = pygame.font.Font(None, 36)
text = font.render("游戏结束,你的得分是:" + str(score), True, (255, 255, 255))
text_rect = text.get_rect()
text_rect.centerx = screen.get_rect().centerx
text_rect.centery = screen.get_rect().centery
screen.blit(text, text_rect)
pygame.display.update()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
```
5. 定义游戏主函数,包括小球和挡板的生成和移动,以及游戏得分的计算
```python
def game():
ball = Ball(300, 0, 10, 5)
paddle = Paddle(250, 380, 100, 10, 5)
score = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
# 按键操作
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
paddle.move_left()
if keys[pygame.K_RIGHT]:
paddle.move_right()
# 小球移动和碰撞检测
ball.move()
if ball.y >= 380 and ball.x >= paddle.x and ball.x <= paddle.x + paddle.width:
score += 1
ball.y = 0
elif ball.y >= 400:
score -= 2
ball.y = 0
if score >= 10:
end_screen(score)
return
# 绘制游戏界面
screen.fill((0, 0, 0))
ball.draw()
paddle.draw()
font = pygame.font.Font(None, 36)
text = font.render("得分:" + str(score), True, (255, 255, 255))
screen.blit(text, (10, 10))
pygame.display.update()
```
6. 最后,在主程序中调用游戏开始界面和游戏主函数
```python
if __name__ == '__main__':
start_screen()
game()
```
完整代码如下:
相关推荐
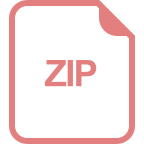












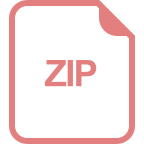
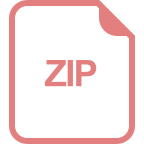