用python将图像二值化,形态学运算,轮廓提取,扣取主体,更换背景图像的代码
时间: 2023-06-06 18:06:37 浏览: 83
以下是使用python进行图像二值化、形态学运算、轮廓提取、扣取主体和更换背景图像的代码:
```python
import cv2
# 读取原始图像
img = cv2.imread("original_image.jpg")
# 进行二值化处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, binary = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 进行形态学处理
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
binary = cv2.dilate(binary, kernel, iterations=2)
# 进行轮廓提取
contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 进行主体扣取
area_threshold = 2000 # 面积阈值
for i, contour in enumerate(contours):
area = cv2.contourArea(contour)
if area > area_threshold:
x, y, w, h = cv2.boundingRect(contour)
roi = img[y:y + h, x:x + w]
cv2.imwrite(f"roi_{i}.jpg", roi)
# 更换背景图像
background = cv2.imread("background_image.jpg")
for i, contour in enumerate(contours):
area = cv2.contourArea(contour)
if area > area_threshold:
x, y, w, h = cv2.boundingRect(contour)
roi = img[y:y + h, x:x + w]
roi_mask = cv2.inRange(binary, 255, 255)
roi_mask = cv2.cvtColor(roi_mask, cv2.COLOR_GRAY2BGR)
background[y:y + h, x:x + w] = cv2.bitwise_and(background[y:y + h, x:x + w], cv2.bitwise_not(roi_mask))
background[y:y + h, x:x + w] += roi
# 显示结果
cv2.imshow("Original Image", img)
cv2.imshow("Binary Image", binary)
cv2.imshow("Background Image", background)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意,以上代码仅为示例代码,具体应用中需要根据实际情况进行调整和优化。
相关推荐
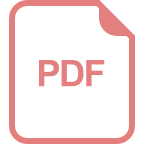
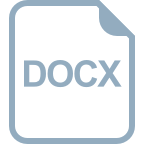
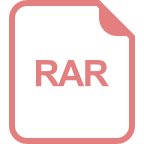














