java查询天气预报
时间: 2024-11-03 18:19:24 浏览: 12
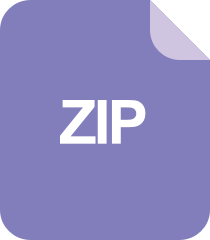
基于java swing的天气预报查询软件
在Java中,查询天气预报通常会通过网络请求API来获取数据。你可以使用第三方库如`org.json`处理JSON数据,或者利用像`retrofit`、`okhttp`这样的HTTP客户端库进行网络通信。以下是一个简单的示例,假设我们有一个公开的天气API:
```java
import org.json.JSONObject;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class WeatherService {
private static final String API_KEY = "your_api_key";
private static final String BASE_URL = "https://api.openweathermap.org/data/2.5/weather";
public JSONObject getWeather(String city) throws Exception {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(BASE_URL + "?q=" + city + "&appid=" + API_KEY)
.build();
try (Response response = client.newCall(request).execute()) {
if (!response.isSuccessful()) throw new IOException("Unexpected code " + response);
String responseBody = response.body().string();
return new JSONObject(responseBody);
}
}
// 使用getWeather()方法获取到的数据,解析并提取所需天气信息
public void displayWeather(JSONObject weatherData) {
try {
int temperature = weatherData.getInt("main.temp");
String description = weatherData.getString("weather")[0].get("description");
System.out.println("当前城市天气:" + description + ", 温度:" + temperature + "K");
} catch (JSONException e) {
e.printStackTrace();
}
}
}
```
在这个例子中,你需要替换`your_api_key`为你从天气服务提供商处获取的实际API密钥。然后通过`displayWeather()`方法来显示获取到的天气信息。
阅读全文
相关推荐
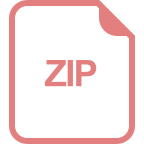
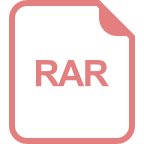
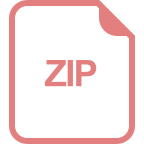
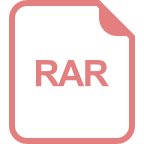
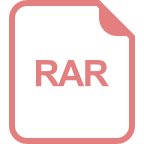
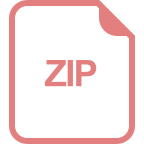
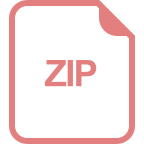
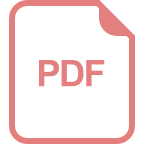
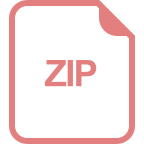
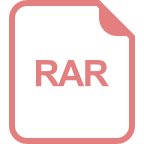
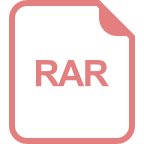
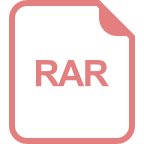
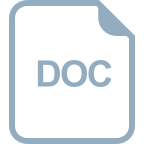
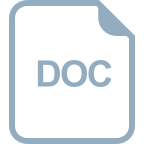

