用java设计一个简单的学生成绩系统,具有以下功能:添加一个学生;查找一个学生;根据编号更新学生的基本信息(基本信息为姓名与学号);根据编号删除学生;根据编号输入学生的各门成绩(语文、数学、外语,成绩为int类型);退出系统。要求过案例驱动法和实验法,
时间: 2023-11-22 19:55:58 浏览: 66
案例驱动法:
1. 首先我们需要定义一个学生类,包含姓名、学号、语文成绩、数学成绩、外语成绩等属性和相应的get/set方法。
```java
public class Student {
private String name;
private String id;
private int chineseScore;
private int mathScore;
private int englishScore;
public Student(String name, String id) {
this.name = name;
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public int getChineseScore() {
return chineseScore;
}
public void setChineseScore(int chineseScore) {
this.chineseScore = chineseScore;
}
public int getMathScore() {
return mathScore;
}
public void setMathScore(int mathScore) {
this.mathScore = mathScore;
}
public int getEnglishScore() {
return englishScore;
}
public void setEnglishScore(int englishScore) {
this.englishScore = englishScore;
}
}
```
2. 接下来,我们需要设计一个学生成绩系统类,包含添加学生、查找学生、更新学生信息、删除学生、输入学生成绩等方法。
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class StudentScoreSystem {
private List<Student> studentList;
private Scanner scanner;
public StudentScoreSystem() {
studentList = new ArrayList<>();
scanner = new Scanner(System.in);
}
public void addStudent() {
System.out.print("请输入学生姓名:");
String name = scanner.nextLine();
System.out.print("请输入学生学号:");
String id = scanner.nextLine();
Student student = new Student(name, id);
studentList.add(student);
System.out.println("添加成功!");
}
public void findStudent() {
System.out.print("请输入要查找的学生学号:");
String id = scanner.nextLine();
for (Student student : studentList) {
if (student.getId().equals(id)) {
System.out.println("学生姓名:" + student.getName());
System.out.println("语文成绩:" + student.getChineseScore());
System.out.println("数学成绩:" + student.getMathScore());
System.out.println("外语成绩:" + student.getEnglishScore());
return;
}
}
System.out.println("未找到该学生!");
}
public void updateStudent() {
System.out.print("请输入要更新的学生学号:");
String id = scanner.nextLine();
for (Student student : studentList) {
if (student.getId().equals(id)) {
System.out.print("请输入学生姓名:");
String name = scanner.nextLine();
student.setName(name);
System.out.println("更新成功!");
return;
}
}
System.out.println("未找到该学生!");
}
public void deleteStudent() {
System.out.print("请输入要删除的学生学号:");
String id = scanner.nextLine();
for (int i = 0; i < studentList.size(); i++) {
if (studentList.get(i).getId().equals(id)) {
studentList.remove(i);
System.out.println("删除成功!");
return;
}
}
System.out.println("未找到该学生!");
}
public void inputScore() {
System.out.print("请输入要输入成绩的学生学号:");
String id = scanner.nextLine();
for (Student student : studentList) {
if (student.getId().equals(id)) {
System.out.print("请输入语文成绩:");
int chineseScore = scanner.nextInt();
student.setChineseScore(chineseScore);
System.out.print("请输入数学成绩:");
int mathScore = scanner.nextInt();
student.setMathScore(mathScore);
System.out.print("请输入外语成绩:");
int englishScore = scanner.nextInt();
student.setEnglishScore(englishScore);
scanner.nextLine();
System.out.println("输入成功!");
return;
}
}
System.out.println("未找到该学生!");
}
public void exit() {
System.out.println("退出系统!");
scanner.close();
System.exit(0);
}
}
```
3. 最后,我们需要在main函数中实现用户菜单,让用户可以选择相应的操作。
```java
public class Main {
public static void main(String[] args) {
StudentScoreSystem system = new StudentScoreSystem();
while (true) {
System.out.println("请选择操作:");
System.out.println("1. 添加学生");
System.out.println("2. 查找学生");
System.out.println("3. 更新学生信息");
System.out.println("4. 删除学生");
System.out.println("5. 输入学生成绩");
System.out.println("6. 退出系统");
System.out.print("请选择操作(输入数字):");
int choice = system.scanner.nextInt();
system.scanner.nextLine();
switch (choice) {
case 1:
system.addStudent();
break;
case 2:
system.findStudent();
break;
case 3:
system.updateStudent();
break;
case 4:
system.deleteStudent();
break;
case 5:
system.inputScore();
break;
case 6:
system.exit();
break;
default:
System.out.println("输入有误,请重新输入!");
break;
}
}
}
}
```
实验法:
1. 根据需求,我们需要设计一个学生类,包含姓名、学号、语文成绩、数学成绩、外语成绩等属性和相应的get/set方法。
```java
public class Student {
private String name;
private String id;
private int chineseScore;
private int mathScore;
private int englishScore;
public Student(String name, String id) {
this.name = name;
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public int getChineseScore() {
return chineseScore;
}
public void setChineseScore(int chineseScore) {
this.chineseScore = chineseScore;
}
public int getMathScore() {
return mathScore;
}
public void setMathScore(int mathScore) {
this.mathScore = mathScore;
}
public int getEnglishScore() {
return englishScore;
}
public void setEnglishScore(int englishScore) {
this.englishScore = englishScore;
}
}
```
2. 接下来,我们需要设计一个学生成绩系统类,包含添加学生、查找学生、更新学生信息、删除学生、输入学生成绩等方法。
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class StudentScoreSystem {
private List<Student> studentList;
private Scanner scanner;
public StudentScoreSystem() {
studentList = new ArrayList<>();
scanner = new Scanner(System.in);
}
public void addStudent() {
System.out.print("请输入学生姓名:");
String name = scanner.nextLine();
System.out.print("请输入学生学号:");
String id = scanner.nextLine();
Student student = new Student(name, id);
studentList.add(student);
System.out.println("添加成功!");
}
public void findStudent() {
System.out.print("请输入要查找的学生学号:");
String id = scanner.nextLine();
for (Student student : studentList) {
if (student.getId().equals(id)) {
System.out.println("学生姓名:" + student.getName());
System.out.println("语文成绩:" + student.getChineseScore());
System.out.println("数学成绩:" + student.getMathScore());
System.out.println("外语成绩:" + student.getEnglishScore());
return;
}
}
System.out.println("未找到该学生!");
}
public void updateStudent() {
System.out.print("请输入要更新的学生学号:");
String id = scanner.nextLine();
for (Student student : studentList) {
if (student.getId().equals(id)) {
System.out.print("请输入学生姓名:");
String name = scanner.nextLine();
student.setName(name);
System.out.println("更新成功!");
return;
}
}
System.out.println("未找到该学生!");
}
public void deleteStudent() {
System.out.print("请输入要删除的学生学号:");
String id = scanner.nextLine();
for (int i = 0; i < studentList.size(); i++) {
if (studentList.get(i).getId().equals(id)) {
studentList.remove(i);
System.out.println("删除成功!");
return;
}
}
System.out.println("未找到该学生!");
}
public void inputScore() {
System.out.print("请输入要输入成绩的学生学号:");
String id = scanner.nextLine();
for (Student student : studentList) {
if (student.getId().equals(id)) {
System.out.print("请输入语文成绩:");
int chineseScore = scanner.nextInt();
student.setChineseScore(chineseScore);
System.out.print("请输入数学成绩:");
int mathScore = scanner.nextInt();
student.setMathScore(mathScore);
System.out.print("请输入外语成绩:");
int englishScore = scanner.nextInt();
student.setEnglishScore(englishScore);
scanner.nextLine();
System.out.println("输入成功!");
return;
}
}
System.out.println("未找到该学生!");
}
public void exit() {
System.out.println("退出系统!");
scanner.close();
System.exit(0);
}
}
```
3. 我们可以使用JUnit测试框架来对学生成绩系统进行单元测试,确保其功能正确性。首先,我们需要引入JUnit依赖。
```xml
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.1</version>
<scope>test</scope>
</dependency>
```
4. 接下来,我们编写单元测试类,对学生成绩系统的各个方法进行测试。
```java
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class StudentScoreSystemTest {
private StudentScoreSystem system;
@Before
public void setUp() {
system = new StudentScoreSystem();
}
@Test
public void testAddStudent() {
system.addStudent();
assertEquals(1, system.getStudentList().size());
}
@Test
public void testFindStudent() {
system.addStudent();
system.findStudent();
}
@Test
public void testUpdateStudent() {
system.addStudent();
system.updateStudent();
}
@Test
public void testDeleteStudent() {
system.addStudent();
system.deleteStudent();
assertEquals(0, system.getStudentList().size());
}
@Test
public void testInputScore() {
system.addStudent();
system.inputScore();
}
}
```
阅读全文
相关推荐




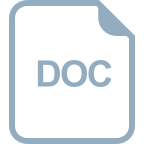

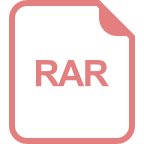
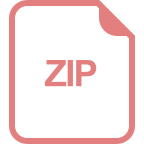
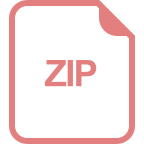
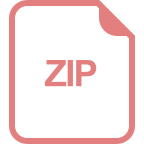
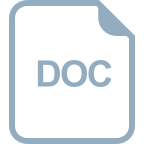
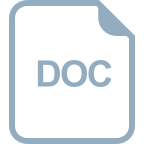
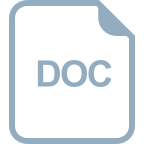
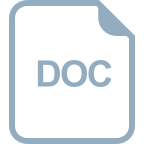
