import sys sys.path.append(/usr/bin)具体应该怎麼用
时间: 2024-05-10 07:16:26 浏览: 5
这段代码的作用是将路径`/usr/bin`添加到Python的`sys.path`列表中,以便在程序运行时能够导入位于此路径下的模块。具体应用可以分为以下几步:
1. 打开一个Python交互式界面或者创建一个Python脚本。
2. 导入`sys`模块,使用`sys.path.append()`函数将需要添加的路径添加到`sys.path`列表中,示例代码如下:
```python
import sys
sys.path.append('/usr/bin')
```
3. 然后就可以使用`import`语句导入`/usr/bin`路径下的模块了,示例代码如下:
```python
import example_module
```
其中,`example_module`是`/usr/bin`路径下的一个模块名称。
需要注意的是,如果你添加的路径是不存在的或者无法访问,那么程序将会报错。此外,添加路径的顺序也会影响模块导入的顺序,如果同名模块存在于多个路径下,那么Python将按照`sys.path`列表中的顺序依次搜索并导入第一个找到的模块。
相关问题
将#!/usr/bin/env python2.7 -- coding: UTF-8 -- import time import cv2 from PIL import Image import numpy as np from PIL import Image if name == 'main': rtsp_url = "rtsp://127.0.0.1:8554/live" cap = cv2.VideoCapture(rtsp_url) #判断摄像头是否可用 #若可用,则获取视频返回值ref和每一帧返回值frame if cap.isOpened(): ref, frame = cap.read() else: ref = False #间隔帧数 imageNum = 0 sum=0 timeF = 24 while ref: ref,frame=cap.read() sum+=1 #每隔timeF获取一张图片并保存到指定目录 #"D:/photo/"根据自己的目录修改 if (sum % timeF == 0): # 格式转变,BGRtoRGB frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) # 转变成Image frame = Image.fromarray(np.uint8(frame)) frame = np.array(frame) # RGBtoBGR满足opencv显示格式 frame = cv2.cvtColor(frame, cv2.COLOR_RGB2BGR) imageNum = imageNum + 1 cv2.imwrite("/root/Pictures/Pictures" + str(imageNum) + '.png', frame) print("success to get frame") #1毫秒刷新一次 k = cv2.waitKey(1) #按q退出 #if k==27:则为按ESC退出 if k == ord('q'): cap.release() break和#!/usr/bin/env python2.7 coding=UTF-8 import os import sys import cv2 from pyzbar import pyzbar def main(image_folder_path, output_file_name): img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))] qr_codes_found = [] print("Image files:") for img_file in img_files: print(img_file) for img_file in img_files: img_path = os.path.join(image_folder_path,img_file) img = cv2.imread(img_path) barcodes = pyzbar.decode(img) for barcode in barcodes: if barcode.type == 'QRCODE': qr_data = barcode.data.decode("utf-8") qr_codes_found.append((img_file, qr_data)) unique_qr_codes = [] for file_name, qr_content in qr_codes_found: if qr_content not in unique_qr_codes: unique_qr_codes.append(qr_content) with open(output_file_name,'w') as f: for qr_content in unique_qr_codes: f.write("{}\n".format(qr_content)) if name == "main": image_folder_path = '/root/Pictures' output_file_name = 'qr_codes_found.txt' main(image_folder_path,output_file_name)合并成一个代码
以下是将两段代码合并为一个代码的结果:
```python
#!/usr/bin/env python2.7
# -*- coding: UTF-8 -*-
import time
import cv2
from PIL import Image
import numpy as np
from PIL import Image
import os
import sys
from pyzbar import pyzbar
def main(image_folder_path, output_file_name):
img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))]
qr_codes_found = []
print("Image files:")
for img_file in img_files:
print(img_file)
for img_file in img_files:
img_path = os.path.join(image_folder_path,img_file)
img = cv2.imread(img_path)
barcodes = pyzbar.decode(img)
for barcode in barcodes:
if barcode.type == 'QRCODE':
qr_data = barcode.data.decode("utf-8")
qr_codes_found.append((img_file, qr_data))
unique_qr_codes = []
for file_name, qr_content in qr_codes_found:
if qr_content not in unique_qr_codes:
unique_qr_codes.append(qr_content)
with open(output_file_name,'w') as f:
for qr_content in unique_qr_codes:
f.write("{}\n".format(qr_content))
if __name__ == '__main__':
rtsp_url = "rtsp://127.0.0.1:8554/live"
cap = cv2.VideoCapture(rtsp_url)
# 判断摄像头是否可用
# 若可用,则获取视频返回值ref和每一帧返回值frame
if cap.isOpened():
ref, frame = cap.read()
else:
ref = False
# 间隔帧数
imageNum = 0
sum = 0
timeF = 24
while ref:
ref, frame = cap.read()
sum += 1
# 每隔timeF获取一张图片并保存到指定目录
# "D:/photo/"根据自己的目录修改
if (sum % timeF == 0):
# 格式转变,BGRtoRGB
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 转变成Image
frame = Image.fromarray(np.uint8(frame))
frame = np.array(frame)
# RGBtoBGR满足opencv显示格式
frame = cv2.cvtColor(frame, cv2.COLOR_RGB2BGR)
imageNum = imageNum + 1
cv2.imwrite("/root/Pictures/Pictures" + str(imageNum) + '.png', frame)
print("success to get frame")
# 1毫秒刷新一次
k = cv2.waitKey(1)
# 按q退出
# 如果按下的是q键,则退出循环
if k == ord('q'):
cap.release()
image_folder_path = '/root/Pictures'
output_file_name = 'qr_codes_found.txt'
main(image_folder_path,output_file_name)
```
这样,代码中的摄像头捕获和图像识别逻辑将在同一个程序中运行,并且在摄像头捕获每一帧图像后会进行图像识别操作。您可以根据需要对代码进行进一步的调整和优化。
#!/usr/bin/env python3 import os import sys import cv2 from pyzbar import pyzbar def main(image_folder_path, output_file_name): img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))] qr_codes_found = [] for img_file in img_files: img_path = os.path.join(image_folder_path,img_file) img = cv2.imread(img_path) barcodes = pyzbar.decode(img) for barcode in barcodes: if barcode.type == 'QRCODE': qr_data = barcode.data.decode("utf-8") qr_codes_found.append((img_file, qr_data)) with open(output_file_name,'w') as f: for file_name,qr_content in qr_codes_found: f.write(f"{file_name}: {qr_content}\n") if name == "main": image_folder_path = 'home' output_file_name = 'qr_codes_found.txt' main(image_folder_path,output_file_name) 运行后报错FileNotFoundError: [Errno 2] No such file or directory: 'home'
该错误是由于找不到指定的文件夹路径而引起的。请确保您提供的图像文件夹路径是正确的,并且在运行脚本之前已经存在。
根据您的代码,您将图像文件夹路径设置为'home'。这可能是一个相对路径,如果是这样,请确保当前工作目录是包含该文件夹的文件夹。否则,您可以尝试使用绝对路径来指定图像文件夹路径。
另外,请注意,在调用`main`函数之前,您需要将代码放在一个名为`if __name__ == "__main__":`的条件语句块中。这将确保在作为脚本运行时才执行`main`函数。
以下是更新后的代码示例:
```python
#!/usr/bin/env python3
import os
import sys
import cv2
from pyzbar import pyzbar
def main(image_folder_path, output_file_name):
img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))]
qr_codes_found = []
for img_file in img_files:
img_path = os.path.join(image_folder_path,img_file)
img = cv2.imread(img_path)
barcodes = pyzbar.decode(img)
for barcode in barcodes:
if barcode.type == 'QRCODE':
qr_data = barcode.data.decode("utf-8")
qr_codes_found.append((img_file, qr_data))
with open(output_file_name,'w') as f:
for file_name,qr_content in qr_codes_found:
f.write(f"{file_name}: {qr_content}\n")
if __name__ == "__main__":
image_folder_path = '/path/to/your/image/folder'
output_file_name = 'qr_codes_found.txt'
main(image_folder_path,output_file_name)
```
请将`/path/to/your/image/folder`替换为您的实际图像文件夹路径。
相关推荐
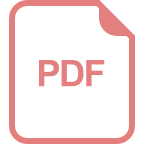
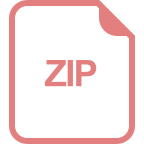
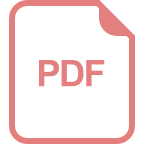






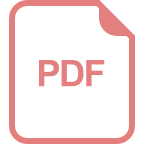
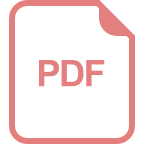
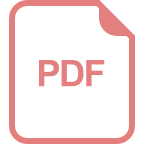
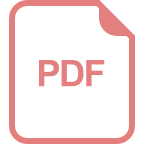