cmp 命令的调用格式
时间: 2023-10-16 13:09:10 浏览: 150
`cmp` 命令用于比较两个文件的内容是否相同,调用格式如下:
```
cmp [OPTION]... FILE1 [FILE2 [SKIP1 [SKIP2]]]
```
其中,`FILE1` 和 `FILE2` 是要比较的两个文件的路径。可以省略 `FILE2`,这样 `cmp` 将会从标准输入中读取内容进行比较。
可用的选项包括:
- `-b` 或 `--print-bytes`:以字节形式输出不同之处的位置。
- `-i BYTES` 或 `--ignore-initial=BYTES`:忽略每个文件中的前面 `BYTES` 字节。
- `-l` 或 `--verbose`:详细输出不同之处的位置。
- `-n BYTES` 或 `--bytes=BYTES`:仅比较每个文件的前面 `BYTES` 字节。
- `-s` 或 `--quiet` 或 `--silent`:不输出任何信息,只返回退出码。
更多选项和用法,请参考 `cmp` 命令的文档或运行 `man cmp` 在终端上查看完整的帮助信息。
相关问题
使用Linux系统调用编写一个完成文件拷贝的C程序。比较拷贝得到的文件与源文件的大小和内容(命令diff,cmp)。
在Linux系统中,你可以使用标准库函数`read`、`write`以及系统调用`open`和`close`来创建一个基本的C程序,用于文件拷贝。以下是简单的步骤和一个示例程序:
首先,打开源文件:
```c
#include <fcntl.h> // 包含文件描述符操作的头文件
int src_fd = open("source_file.txt", O_RDONLY); // O_RDONLY表示只读模式
```
接着,准备目标文件并打开它以便写入:
```c
char dest_buf[BUFSIZ]; // 存储临时缓冲区
int dest_fd = open("destination_file.txt", O_WRONLY | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR); // O_WRONLY表示写入,O_CREAT如果不存在则创建,O_TRUNC会清空文件内容
```
然后,开始逐块读取源文件并写入到目标文件:
```c
while (true) {
ssize_t read_size = read(src_fd, dest_buf, sizeof(dest_buf));
if (read_size <= 0)
break; // 如果读取结束或者出错,则跳出循环
write(dest_fd, dest_buf, read_size); // 写入目标文件
}
```
关闭两个文件描述符:
```c
close(src_fd);
close(dest_fd);
```
最后,你可以使用`cmp`命令比较两个文件的内容,如果它们相等,`cmp`通常会返回0;而使用`du -b`命令比较文件大小:
```sh
size_source = du -b source_file.txt | cut -f1;
size_destination = du -b destination_file.txt | cut -f1;
if (size_source == size_destination && !cmp source_file.txt destination_file.txt) {
printf("文件大小一致且内容相同.\n");
} else {
printf("文件大小或内容有差异.\n");
}
```
使用Linux系统调用编写一个完成文件拷贝的C程序。比较拷贝得到的文件与源文件的大小和内容(命令diff,cmp)。
在Linux环境下,你可以使用C语言通过系统调用`read()`和`write()`来直接操作文件,或者使用标准库函数`fopen()`, `fwrite()`, 和`fclose()`。这里是一个基本的例子,展示如何创建一个简单的C程序,用于读取源文件并复制到目标文件,然后使用`diff`和`cmp`命令对比它们的内容:
```c
#include <stdio.h>
#include <fcntl.h> // for open and close file descriptors
#include <unistd.h> // for read and write system calls
#define BUFFER_SIZE 4096 // 文件块大小
void copy_file(char* src, char* dst) {
int src_fd = open(src, O_RDONLY); // 打开源文件
int dst_fd = creat(dst, S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH); // 创建目标文件
if (src_fd == -1 || dst_fd == -1) {
perror("Error opening files");
return;
}
char buffer[BUFFER_SIZE];
ssize_t bytes_read;
while ((bytes_read = read(src_fd, buffer, BUFFER_SIZE)) > 0) {
write(dst_fd, buffer, bytes_read); // 复制数据
}
close(src_fd);
close(dst_fd);
if (bytes_read == -1) {
perror("Error reading or writing");
}
}
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s <source_file> <destination_file>\n", argv[0]);
return 1;
}
char* src = argv[1];
char* dst = argv[2];
copy_file(src, dst);
// 比较文件
FILE* src_file = fopen(src, "r");
FILE* dst_file = fopen(dst, "r");
if (!src_file || !dst_file) {
perror("Error opening comparison files");
return 1;
}
size_t source_size = 0, dest_size = 0;
fseek(src_file, 0L, SEEK_END);
source_size = ftell(src_file);
rewind(src_file);
fseek(dst_file, 0L, SEEK_END);
dest_size = ftell(dst_file);
rewind(dst_file);
fclose(src_file);
fclose(dst_file);
if (source_size == dest_size) {
printf("Files have the same size.\n");
} else {
printf("Files have different sizes.\n");
}
// 使用diff命令比较文件内容(假设已经安装了diff)
system("diff -q " src " " dst); // 如果返回0,表示两文件内容一致
// 使用cmp命令比较文件内容(假设已经安装了cmp)
// system("cmp -silent " src " " dst); // 如果返回0,表示两文件内容一致
return 0;
}
```
运行这个程序前,请确保`diff`和`cmp`命令已经在你的路径中可用。如果文件大小和内容相同,上述脚本会打印相应的信息,并显示文件内容是否一致。
阅读全文
相关推荐
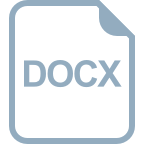
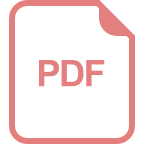
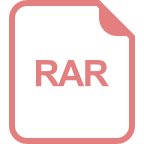
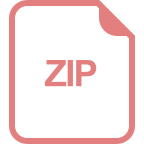
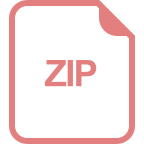
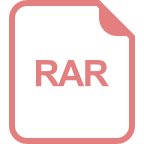
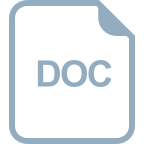
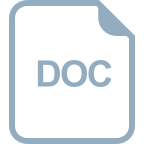
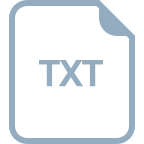
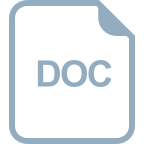
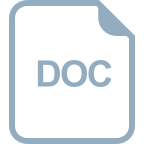
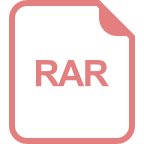
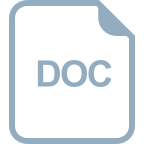
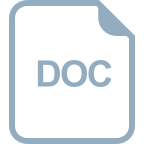
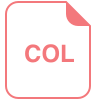
