OC layer或者NSView上的图层保存到NSImage
时间: 2023-09-05 12:07:07 浏览: 51
将OC layer或NSView上的图层保存到NSImage可以使用以下方法:
1. 首先创建一个NSBitmapImageRep对象
```
NSBitmapImageRep *bitmapRep = [self bitmapImageRepForCachingDisplayInRect:self.bounds];
```
2. 将OC layer或者NSView上的图层绘制到bitmapRep中
```
[self cacheDisplayInRect:self.bounds toBitmapImageRep:bitmapRep];
```
3. 创建一个NSImage对象并将bitmapRep添加到其中
```
NSImage *image = [[NSImage alloc] initWithSize:self.bounds.size];
[image addRepresentation:bitmapRep];
```
4. 现在你就可以将这个NSImage对象保存到磁盘或者进行其他操作了。
```
NSData *imageData = [image TIFFRepresentation];
[imageData writeToFile:@"path/to/image.png" atomically:YES];
```
注意:这里的self指代的是OC layer或者NSView。
相关问题
layer或者NSView上的图层保存到NSImage
在 macOS 中,可以使用以下代码将图层保存为 NSImage:
```swift
// 将图层转换为位图上下文
let bitmapRep = layer.bitmapImageRepForCachingDisplay(in: layer.bounds)!
layer.cacheDisplay(in: layer.bounds, to: bitmapRep)
// 创建 NSImage
let image = NSImage(size: layer.bounds.size)
image.addRepresentation(bitmapRep)
```
其中,`layer` 是你要保存为图像的图层。该代码将图层转换为位图上下文,并将其缓存。然后,将位图添加到新的 NSImage 对象中。最终,你可以将该图像保存到磁盘或在应用程序中显示。
oc之mac坐标转换
在 macOS 中,有多种方式进行坐标转换。以下是其中两种常见的方法:
1. 使用坐标转换函数
在 macOS 中,可以使用以下函数将一个坐标点从一个坐标系转换到另一个坐标系:
```objc
NSPoint NSConvertPoint(NSPoint aPoint, NSRect fromRect, NSRect toRect);
```
其中,`aPoint` 是需要转换的坐标点,`fromRect` 是源坐标系(通常是屏幕坐标系),`toRect` 是目标坐标系(通常是视图坐标系)。例如,要将屏幕上的坐标点转换为视图上的坐标点,可以这样写:
```objc
NSPoint screenPoint = {100, 100}; // 屏幕坐标系
NSRect viewFrame = [self.view frame]; // 视图坐标系
NSPoint viewPoint = NSConvertPoint(screenPoint, nil, viewFrame); // 转换坐标
```
2. 使用坐标转换矩阵
另一种常见的方式是使用坐标转换矩阵。在 macOS 中,可以使用以下方法创建一个坐标转换矩阵:
```objc
NSAffineTransform *transform = [NSAffineTransform transform];
[transform scaleXBy:scaleX yBy:scaleY];
[transform rotateByDegrees:rotation];
[transform translateXBy:translateX yBy:translateY];
```
其中,`scaleX` 和 `scaleY` 是缩放因子,`rotation` 是旋转角度,`translateX` 和 `translateY` 是平移量。例如,要将屏幕上的坐标点转换为视图上的坐标点,可以这样写:
```objc
NSAffineTransform *transform = [NSAffineTransform transform];
[transform translateXBy:-viewFrame.origin.x yBy:-viewFrame.origin.y]; // 将视图坐标系移到原点
[transform concat]; // 应用坐标转换矩阵
NSPoint screenPoint = {100, 100}; // 屏幕坐标系
NSPoint viewPoint = [self.view convertPoint:screenPoint fromView:nil]; // 转换坐标
```
这里使用了 `NSView` 类的 `convertPoint:fromView:` 方法,将屏幕上的坐标点转换为视图上的坐标点。
相关推荐
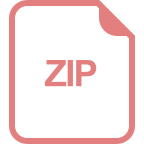











