C++中set集合用法
时间: 2024-09-26 11:14:41 浏览: 36
在C++中,`std::set`是一种关联容器,它存储了一组唯一元素(不允许有重复),并按照一定的顺序(默认是升序,可以自定义比较函数)进行排列。`set`是基于红黑树实现的,提供了插入、删除、查找等操作,时间复杂度通常接近O(log n)。
以下是使用`std::set`的一些基本步骤:
1. 包含头文件:
```cpp
#include <iostream>
#include <set>
```
2. 创建set实例:
```cpp
std::set<int> mySet; // 存储整数的无序集合
```
如果你想存储自定义类型的元素,需要提供相应的比较函数:
```cpp
struct MyData {
// 定义数据成员及比较规则
};
bool compare(MyData const& a, MyData const& b) {
// 比较两个对象的规则
}
std::set<MyData, decltype(&compare)> myCustomSet(compare); // 使用自定义比较函数
```
3. 插入元素:
```cpp
mySet.insert(5); // 添加整数
myCustomSet.insert({ /* 自定义对象 */ }); // 或者添加自定义对象
```
4. 查找元素(如果存在返回迭代器,不存在则返回end()):
```cpp
auto it = mySet.find(5);
if (it != mySet.end()) {
std::cout << "Found " << *it << std::endl;
}
```
5. 删除元素:
```cpp
mySet.erase(it); // 如果找到了元素
```
6. 遍历集合:
```cpp
for (const auto& elem : mySet) {
std::cout << elem << " ";
}
```
阅读全文
相关推荐
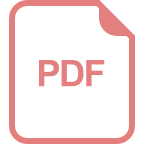
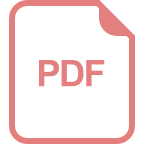
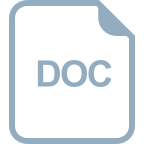















