Linux C 进程和线程怎么创建和销毁
时间: 2024-10-11 20:01:54 浏览: 33
在Linux C语言中,创建进程和线程可以分别通过`fork()`、`execve()`函数以及线程库如pthread创建。
**创建进程:**
1. **Fork()函数** - 使用`fork()`创建新进程。如果调用成功,父进程返回0,子进程返回新的进程ID;父进程返回非零值表示出错。示例:
```c
pid_t pid = fork();
if (pid == 0) {
// 子进程
} else if (pid > 0) {
// 父进程
} else {
perror("fork error");
}
```
**创建线程:**
1. **pthread_create()函数** - 使用POSIX线程库,需要包含`#include <pthread.h>`。首先创建一个线程结构体,然后调用`pthread_create()`。例如:
```c
pthread_t thread_id;
pthread_create(&thread_id, NULL, your_thread_function, arg);
```
其中`your_thread_function`是线程执行的函数指针,`arg`是传给该函数的参数。
**销毁进程或线程:**
1. **Exit()函数** - 在子进程中,可以用`exit()`或`_exit()`来终止并结束进程。
```c
exit(EXIT_SUCCESS); // 正常退出
_exit(EXIT_FAILURE); // 异常退出
```
2. **pthread_exit()函数** - 对于线程,使用`pthread_exit()`终止线程并可能传递一个状态值给主线程。
```c
pthread_exit(NULL); // 结束线程,状态由pthread_join处理
```
3. **Joining threads** - 主线程可以通过`pthread_join()`等待子线程执行完毕再继续。
```c
pthread_join(thread_id, NULL);
```
阅读全文
相关推荐
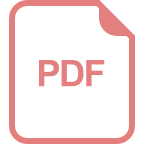
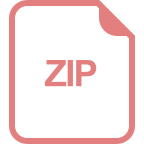
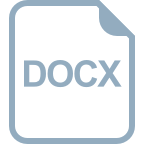






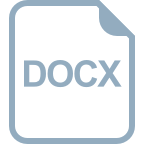
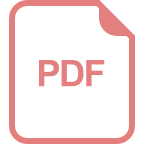
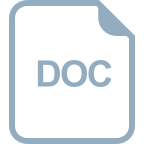
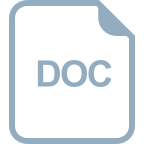
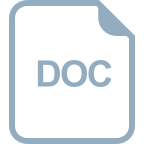




