python用opencv计算图片的侧脸角度并保存侧量出角度的图片
时间: 2023-09-23 13:05:45 浏览: 227
### 回答1:
要计算图片的侧脸角度,可以使用OpenCV库中的人脸检测模块和姿态估计模块。下面是一个基本的代码示例:
```python
import cv2
# 加载人脸检测器和姿态估计器
detector = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 加载图片
img = cv2.imread('test.jpg')
# 灰度化
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 人脸检测
faces = detector.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30), flags=cv2.CASCADE_SCALE_IMAGE)
# 遍历检测到的人脸
for (x, y, w, h) in faces:
# 提取人脸区域
face_img = img[y:y + h, x:x + w].copy()
# 姿态估计
shape = predictor(gray, dlib.rectangle(x, y, x + w, y + h))
angles = face_utils.angle_between_3_points(shape.part(45), shape.part(36), shape.part(48))
# 在图片上绘制角度值
cv2.putText(img, "Angle: {:.2f}".format(angles), (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 保存角度值的图片
cv2.imwrite("angle_{}.jpg".format(int(angles)), face_img)
```
解释一下上面的代码:
1. 首先加载人脸检测器和姿态估计器。
2. 加载要处理的图片并将其灰度化。
3. 使用人脸检测器检测出图片中的人脸区域。
4. 遍历每个检测到的人脸,提取出人脸区域。
5. 使用姿态估计器估计出人脸的角度,并在原始图片上绘制出角度值。
6. 保存角度值的图片。
需要注意的一点是,上面的代码中使用的姿态估计器是基于dlib库的,需要先安装dlib库。另外,上面的代码只能处理单张图片,如果要处理多张图片,需要将代码放到一个循环中,遍历所有的图片。
### 回答2:
使用Python的OpenCV库可以方便地计算图片的侧脸角度并保存侧量出角度的图片。
首先,导入相应的库和模块:
```python
import cv2
import dlib
```
然后,加载并读取需要处理的图片:
```python
image = cv2.imread("image.jpg")
```
接着,使用dlib库中的面部分类器模型来检测人脸:
```python
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
faces = detector(gray)
```
之后,遍历检测到的人脸,并获取其侧脸角度:
```python
for face in faces:
landmarks = predictor(gray, face)
left_eye = (landmarks.part(36).x, landmarks.part(36).y)
right_eye = (landmarks.part(45).x, landmarks.part(45).y)
angle = -1 * np.arctan((right_eye[1] - left_eye[1]) / (right_eye[0] - left_eye[0])) * 180 / np.pi
rotated = cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE)
cv2.putText(rotated, "Angle: {:.2f}".format(angle), (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0, 0, 255), 2)
cv2.imwrite("rotated_image.jpg", rotated)
```
最后,保存旋转后的图片,其中角度信息已添加到图片上。
以上就是使用Python和OpenCV库计算图片的侧脸角度并保存侧量出角度的图片的步骤,通过以上代码可以实现该功能。
### 回答3:
使用Python和OpenCV计算图片的侧脸角度并保存侧测出角度的图片可以通过以下步骤完成:
1. 导入所需的库:
```python
import cv2
import dlib
```
2. 加载人脸识别模型和侧脸检测器:
```python
predictor_path = 'path/to/shape_predictor_68_face_landmarks.dat' # dlib预测器的路径
detector = dlib.get_frontal_face_detector() # 获取人脸检测器
predictor = dlib.shape_predictor(predictor_path) # 获取68点模型
```
3. 加载图片并进行灰度转换:
```python
image_path = 'path/to/image.jpg' # 图片的路径
image = cv2.imread(image_path)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
4. 检测侧脸并计算角度:
```python
faces = detector(gray)
for face in faces:
landmarks = predictor(gray, face)
x_left = landmarks.part(0).x
y_left = landmarks.part(0).y
x_right = landmarks.part(16).x
y_right = landmarks.part(16).y
angle = -1 * math.degrees(math.atan((y_right - y_left) / (x_right - x_left)))
```
5. 旋转图片:
```python
(rows, cols) = image.shape[:2]
M = cv2.getRotationMatrix2D((cols / 2, rows / 2), angle, 1)
rotated = cv2.warpAffine(image, M, (cols, rows))
```
6. 保存旋转后的图片:
```python
output_path = 'path/to/output.jpg' # 输出图片的路径
cv2.imwrite(output_path, rotated)
```
以上代码将加载图片并使用OpenCV和dlib定位并测量侧脸角度,然后将其旋转并保存在指定的输出路径中。请确保安装了所需的库和模型文件,并替换代码中的路径和文件名为你自己的图片和模型。
阅读全文
相关推荐
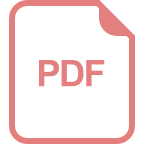


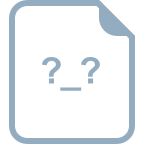
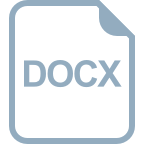
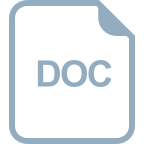


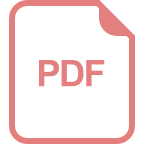
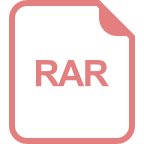
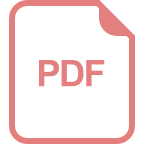
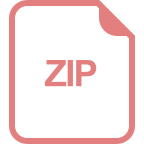
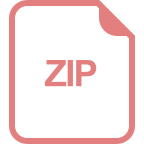
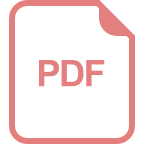
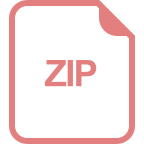
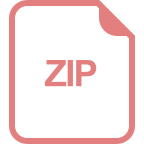
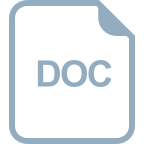