如何在c语言中用scanf函数输出hello
时间: 2024-03-12 12:48:52 浏览: 27
很抱歉,您的问题有点不清楚。如果您是想要在C语言中使用scanf函数输入字符串"hello",可以使用以下代码:
```c
char str[10];
scanf("%s", str);
printf("%s", str);
```
如果您是想要在C语言中使用scanf函数输出字符串"hello",则是不可能的。scanf函数是用于输入数据的函数,而不是输出数据的函数。如果您想要输出字符串"hello",可以使用以下代码:
```c
printf("hello");
```
希望能够帮到您。
相关问题
用scanf函数输出Hello
要使用scanf函数输出"Hello",您可以按照以下步骤进行:
1. 使用scanf函数读取一个字符串,可以使用`%s`格式控制符,将读取到的字符串存储在一个字符数组中。
2. 将字符数组中的第一个字符转换为大写字母,使用toupper函数实现。
3. 使用printf函数输出转换后的字符串。
下面是示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[10];
printf("请输入一个字符串:");
scanf("%s", str);
str[0] = toupper(str[0]);
printf("%s\n", str);
return 0;
}
```
在运行程序时,您需要在命令行输入一个字符串,程序会将其转换为大写字母并输出。例如,如果您输入 "hello",程序会输出 "Hello"。
c语言合法scanf函数调用
在C语言中,合法的scanf函数调用需要满足以下条件:
1. 函数名为scanf。
2. 函数调用的格式为scanf("格式控制字符串", 参数列表)。
3. 格式控制字符串指定了输入的格式,例如"%d"表示输入一个整数,"%f"表示输入一个浮点数,"%s"表示输入一个字符串等。
4. 参数列表中按照格式控制字符串的要求提供了相应类型的变量地址,用于接收输入的值。
例如,合法的scanf函数调用可以是:
```c
int num;
scanf("%d", &num);
```
这个调用表示从标准输入中读取一个整数,并将其存储到变量num中。
相关推荐
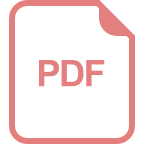
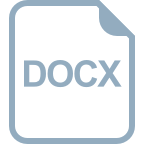
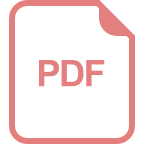
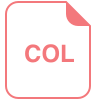
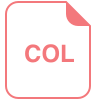
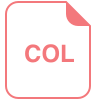
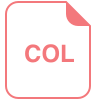
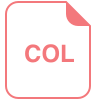







