给java调用的actionPerformed方法中添加一个可以发送文件的功能
时间: 2024-02-01 07:13:49 浏览: 129
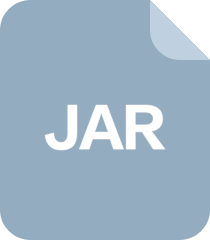
聊天室java可以实现传送文件功能

要实现在Java中添加一个可以发送文件的功能,可以使用Java的Socket编程来实现。以下是一个简单的示例代码:
```java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.net.Socket;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
public class SendFileActionListener implements ActionListener {
private Socket socket;
public SendFileActionListener(Socket socket) {
this.socket = socket;
}
@Override
public void actionPerformed(ActionEvent e) {
JFrame parent = new JFrame();
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(parent);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try {
FileInputStream fis = new FileInputStream(file);
DataOutputStream dos = new DataOutputStream(socket.getOutputStream());
// 发送文件名和文件长度
dos.writeUTF(file.getName());
dos.writeLong(file.length());
// 发送文件内容
byte[] buffer = new byte[4096];
int read = 0;
while ((read = fis.read(buffer)) > 0) {
dos.write(buffer, 0, read);
}
fis.close();
dos.flush();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
}
```
在这个示例中,我们创建了一个名为SendFileActionListener的ActionListener类,它接收一个Socket对象作为构造函数的参数。在actionPerformed方法中,我们首先使用JFileChooser来让用户选择要发送的文件。然后,我们打开这个文件并使用DataOutputStream将文件名和文件长度发送到服务器端。接着,我们使用一个循环来读取文件内容并将其发送到服务器端。最后,我们关闭文件输入流和数据输出流,以及刷新数据输出流。
在主程序中,我们可以将这个ActionListener添加到一个按钮或菜单项中,以便用户可以点击来触发文件发送操作。例如:
```java
Socket socket = new Socket("localhost", 8080);
SendFileActionListener sendFileActionListener = new SendFileActionListener(socket);
JButton sendButton = new JButton("Send File");
sendButton.addActionListener(sendFileActionListener);
```
在这个示例中,我们创建了一个名为socket的Socket对象,它连接到本地主机的8080端口。然后,我们创建了一个名为sendButton的JButton对象,并将SendFileActionListener添加为其ActionListener。这样,当用户点击sendButton时,就会触发文件发送操作。
阅读全文
相关推荐
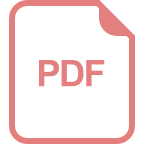

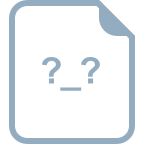
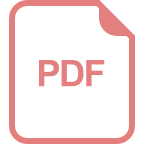
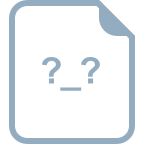
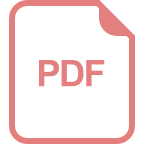
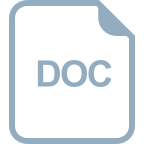
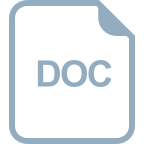
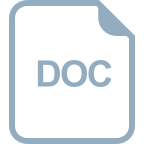
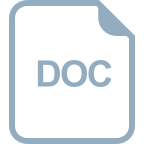
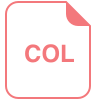
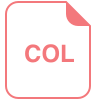
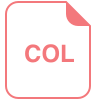
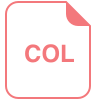