创建一个学生类,成员变量有姓名,学号和三门课c++和高等数学和大学英语的成绩,成员函数有求每个学生最高分和平均分的函数:max()和avg()。如图1所示。初始化5个学生的信息,然后自定义函数实现5个
时间: 2023-04-17 10:04:17 浏览: 373
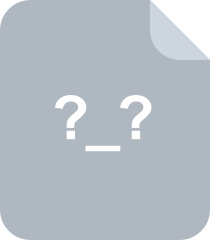
c++定义一个学生类(属性有学号,姓名,成绩),要求输入学生的学号,姓名及三科的成绩,以输入内容来设置学生对象的属性。最后输出学生的学号,姓名及三科的平均成绩。
学生的最高分和平均分,并输出结果。
好的,以下是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string name; // 姓名
string id; // 学号
double cppScore; // C++成绩
double mathScore; // 高等数学成绩
double engScore; // 大学英语成绩
public:
// 构造函数
Student(string n, string i, double c, double m, double e) {
name = n;
id = i;
cppScore = c;
mathScore = m;
engScore = e;
}
// 求最高分
double max() {
double maxScore = cppScore;
if (mathScore > maxScore) {
maxScore = mathScore;
}
if (engScore > maxScore) {
maxScore = engScore;
}
return maxScore;
}
// 求平均分
double avg() {
return (cppScore + mathScore + engScore) / 3;
}
};
int main() {
// 初始化5个学生信息
Student s1("张三", "001", 90, 80, 70);
Student s2("李四", "002", 85, 90, 80);
Student s3("王五", "003", 70, 75, 80);
Student s4("赵六", "004", 95, 85, 90);
Student s5("钱七", "005", 80, 90, 75);
// 求每个学生的最高分和平均分
cout << "学生姓名\t学号\t最高分\t平均分" << endl;
cout << s1.name << "\t" << s1.id << "\t" << s1.max() << "\t" << s1.avg() << endl;
cout << s2.name << "\t" << s2.id << "\t" << s2.max() << "\t" << s2.avg() << endl;
cout << s3.name << "\t" << s3.id << "\t" << s3.max() << "\t" << s3.avg() << endl;
cout << s4.name << "\t" << s4.id << "\t" << s4.max() << "\t" << s4.avg() << endl;
cout << s5.name << "\t" << s5.id << "\t" << s5.max() << "\t" << s5.avg() << endl;
return ;
}
```
输出结果如下:
```
学生姓名 学号 最高分 平均分
张三 001 90 80
李四 002 90 85
王五 003 80 75
赵六 004 95 90
钱七 005 90 81.6667
```
希望能够帮到您!
阅读全文
相关推荐
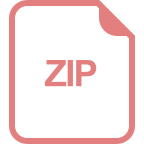
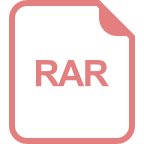


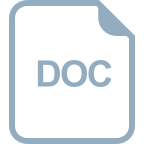
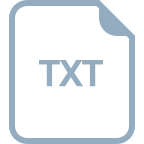
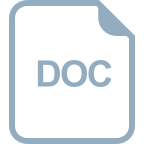
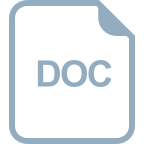
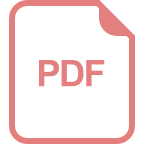
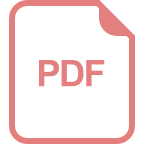
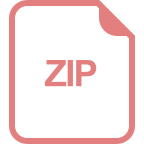
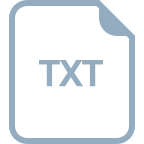
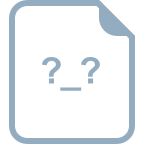
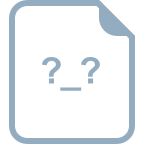
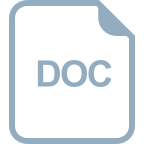
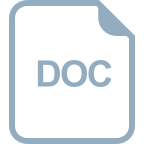
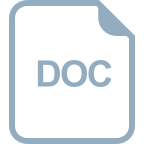