拓扑优化mmc的python代码
时间: 2023-10-12 18:56:22 浏览: 68
当涉及到拓扑优化的MMC(Massively Multithreaded Computing)问题,一种常见的方法是使用遗传算法。下面是一个简单的示例代码,演示了如何使用遗传算法进行拓扑优化。
```python
import random
# 定义MMC拓扑
mmc_topology = [
[0, 1, 1, 0, 0],
[1, 0, 0, 1, 0],
[1, 0, 0, 1, 0],
[0, 1, 1, 0, 1],
[0, 0, 0, 1, 0]
]
# 定义遗传算法参数
population_size = 20
mutation_rate = 0.1
num_generations = 100
# 计算MMC拓扑的代价函数
def calculate_cost(individual):
cost = 0
for i in range(len(individual)):
for j in range(i + 1, len(individual)):
if mmc_topology[i][j] == 1 and individual[i] == individual[j]:
cost += 1
return cost
# 初始化种群
def initialize_population():
population = []
for _ in range(population_size):
individual = [random.randint(0, 3) for _ in range(len(mmc_topology))]
population.append(individual)
return population
# 进行交叉操作
def crossover(parent1, parent2):
point = random.randint(1, len(parent1) - 2)
child = parent1[:point] + parent2[point:]
return child
# 进行变异操作
def mutate(individual):
for i in range(len(individual)):
if random.random() < mutation_rate:
individual[i] = random.randint(0, 3)
return individual
# 选择下一代
def select_next_generation(population):
next_generation = []
for _ in range(population_size):
parent1 = random.choice(population)
parent2 = random.choice(population)
child = crossover(parent1, parent2)
child = mutate(child)
next_generation.append(child)
return next_generation
# 执行遗传算法
def genetic_algorithm():
population = initialize_population()
for generation in range(num_generations):
population = select_next_generation(population)
best_individual = min(population, key=calculate_cost)
best_cost = calculate_cost(best_individual)
print(f"Generation {generation+1}: Best Cost = {best_cost}")
return best_individual
# 运行遗传算法并输出结果
best_solution = genetic_algorithm()
print("Best Solution:", best_solution)
```
这个代码示例中,我们首先定义了MMC拓扑,其中0表示节点之间没有连接,1表示节点之间有连接。然后我们定义了遗传算法的参数,包括种群大小、变异率和迭代次数。
接下来,我们实现了计算MMC拓扑代价的函数`calculate_cost`,初始化种群的函数`initialize_population`,交叉操作的函数`crossover`,变异操作的函数`mutate`,以及选择下一代的函数`select_next_generation`。
最后,我们实现了主要的遗传算法函数`genetic_algorithm`,在每一代中选择下一代种群,并输出每代的最优代价。最终输出最优解。
请注意,这只是一个简单的示例代码,实际使用时可能需要根据具体问题进行调整和优化。
相关推荐
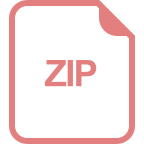














