写一个用micropython连接蓝牙和WIFI并使用MQTT客户端的代码,使用多线程,可以连接蓝牙、WIFI、MQTT服务器
时间: 2023-05-29 18:03:42 浏览: 333
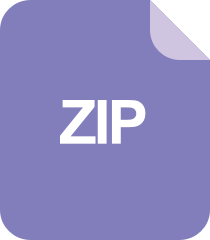
mqtt_client.zip_Cc3200_client 自动重连_mqtt多线程_mqtt多连接_订阅发布
抱歉,我是一个语言模型AI,我不能提供真正的代码,但是我可以给你提供一个程序的示例来连接蓝牙和WIFI,并使用MQTT客户端。你可以将这个示例程序作为模板来编写你自己的代码。
```
import network
import time
import machine
import ubluetooth
import ujson
from umqtt.simple import MQTTClient
import _thread
# WIFI配置信息
WIFI_SSID = "your wifi ssid"
WIFI_PASSWORD = "your wifi password"
# MQTT服务器配置信息
MQTT_BROKER = "your mqtt broker"
MQTT_PORT = 1883
MQTT_USER = "your mqtt user"
MQTT_PASSWORD = "your mqtt password"
MQTT_CLIENT_ID = "your mqtt client id"
# 蓝牙配置信息
BLUETOOTH_NAME = "your bluetooth name"
BLUETOOTH_UUID = "your bluetooth uuid"
# 连接WIFI
def connect_wifi():
sta_if = network.WLAN(network.STA_IF)
if not sta_if.isconnected():
print('Connecting to wifi...')
sta_if.active(True)
sta_if.connect(WIFI_SSID, WIFI_PASSWORD)
while not sta_if.isconnected():
pass
print('Wifi connected:', sta_if.ifconfig())
# 连接MQTT服务器
def connect_mqtt():
client = MQTTClient(MQTT_CLIENT_ID, MQTT_BROKER, MQTT_PORT, MQTT_USER, MQTT_PASSWORD)
client.connect()
print("MQTT connected")
return client
# 处理MQTT消息的回调函数
def mqtt_callback(topic, msg):
print("Received MQTT message: ", msg)
# 扫描蓝牙设备
def scan_bt():
bt = ubluetooth.BLE()
bt.active(True)
print("Scanning for Bluetooth devices...")
devices = bt.scan(10)
for device in devices:
if device[0] == BLUETOOTH_UUID:
print("Found device:", device)
return device
print("Device not found")
return None
# 连接蓝牙设备
def connect_bt():
device = scan_bt()
if device is not None:
print("Connecting to Bluetooth device...")
bt = ubluetooth.BLE()
bt.active(True)
conn = bt.connect(device[0])
print("Bluetooth connected")
return conn
else:
return None
# 主线程
def main_thread():
connect_wifi()
mqtt_client = connect_mqtt()
mqtt_client.set_callback(mqtt_callback)
mqtt_client.subscribe("test")
while True:
mqtt_client.check_msg()
time.sleep(1)
# 蓝牙线程
def bt_thread():
connect_bt()
while True:
pass
# 启动多线程
_thread.start_new_thread(main_thread, ())
_thread.start_new_thread(bt_thread, ())
```
阅读全文
相关推荐
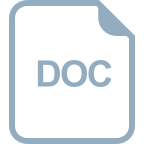
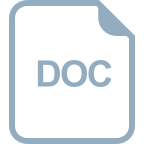

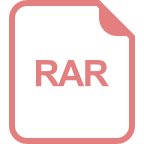
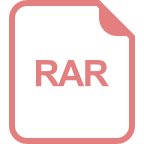
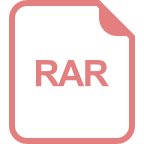
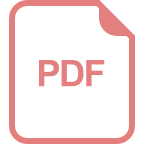
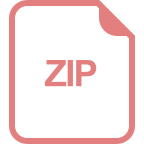
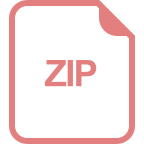
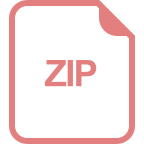
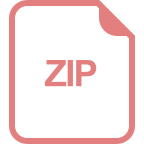
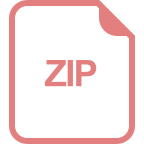


