飞机订票系统c语言课程
时间: 2023-11-12 20:07:37 浏览: 144
飞机订票系统是一个比较典型的信息管理系统,可以使用C语言来实现。以下是一个简单的飞机订票系统的代码示例,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_FLIGHTS 10
#define MAX_NAME_LEN 50
typedef struct {
char name[MAX_NAME_LEN];
int id;
int capacity;
int booked;
} Flight;
Flight flights[MAX_FLIGHTS];
int num_flights = 0;
void add_flight() {
if (num_flights >= MAX_FLIGHTS) {
printf("Sorry, the maximum number of flights has been reached.\n");
return;
}
Flight f;
printf("Enter flight name: ");
scanf("%s", f.name);
printf("Enter flight ID: ");
scanf("%d", &f.id);
printf("Enter flight capacity: ");
scanf("%d", &f.capacity);
f.booked = 0;
flights[num_flights++] = f;
}
void list_flights() {
printf("Flight Name\tFlight ID\tCapacity\tBooked\n");
for (int i = 0; i < num_flights; i++) {
Flight f = flights[i];
printf("%s\t\t%d\t\t%d\t\t%d\n", f.name, f.id, f.capacity, f.booked);
}
}
void book_flight() {
int id;
printf("Enter flight ID: ");
scanf("%d", &id);
int i;
for (i = 0; i < num_flights; i++) {
if (flights[i].id == id) {
break;
}
}
if (i == num_flights) {
printf("Sorry, no flight found with ID %d.\n", id);
return;
}
Flight *f = &flights[i];
if (f->booked >= f->capacity) {
printf("Sorry, the flight is fully booked.\n");
return;
}
printf("Enter passenger name: ");
scanf("%s", f->name);
f->booked++;
printf("Booking successful!\n");
}
int main() {
int choice;
while (1) {
printf("1. Add Flight\n");
printf("2. List Flights\n");
printf("3. Book Flight\n");
printf("4. Quit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_flight();
break;
case 2:
list_flights();
break;
case 3:
book_flight();
break;
case 4:
exit(0);
default:
printf("Invalid choice\n");
}
}
return 0;
}
```
这个示例实现了添加航班、列出航班列表、预定航班等功能。你可以根据需要进行修改和扩展。
阅读全文
相关推荐


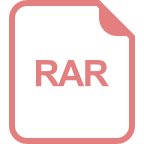





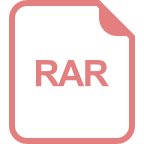

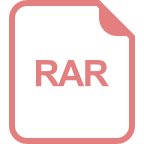