用C语言写一个多产品货物入库出库的代码
时间: 2024-05-13 16:15:44 浏览: 11
以下是一个简单的多产品货物入库出库的C语言代码:
```c
#include <stdio.h>
#define MAX_PRODUCTS 10 // 最大产品数
#define MAX_NAME_LENGTH 20 // 产品名称最大长度
// 产品结构体
typedef struct product {
char name[MAX_NAME_LENGTH]; // 产品名称
int quantity; // 产品数量
} Product;
// 全部产品数组
Product products[MAX_PRODUCTS];
// 添加产品
void addProduct(char *name, int quantity) {
for(int i = 0; i < MAX_PRODUCTS; i++) {
// 查找空闲位置
if(products[i].name[0] == '\0') {
// 添加产品
strcpy(products[i].name, name);
products[i].quantity = quantity;
printf("产品 %s 已添加到仓库中\n", name);
return;
}
}
printf("仓库已满,无法添加产品\n");
}
// 移除产品
void removeProduct(char *name, int quantity) {
for(int i = 0; i < MAX_PRODUCTS; i++) {
// 查找匹配的产品
if(strcmp(products[i].name, name) == 0) {
// 检查数量是否足够
if(products[i].quantity >= quantity) {
// 移除产品
products[i].quantity -= quantity;
printf("从仓库中移除了 %d 个 %s\n", quantity, name);
return;
} else {
printf("%s 的数量不足,无法移除\n", name);
return;
}
}
}
printf("仓库中没有 %s 这个产品\n", name);
}
// 显示仓库中的所有产品
void showProducts() {
printf("仓库中的产品:\n");
for(int i = 0; i < MAX_PRODUCTS; i++) {
if(products[i].name[0] != '\0') {
printf("%d. %s (数量:%d)\n", i+1, products[i].name, products[i].quantity);
}
}
}
int main() {
int choice;
while(1) {
printf("\n请选择操作:\n");
printf("1. 添加产品\n");
printf("2. 移除产品\n");
printf("3. 显示仓库中的所有产品\n");
printf("4. 退出\n");
printf("请选择:");
scanf("%d", &choice);
switch(choice) {
case 1:
// 添加产品
char name[MAX_NAME_LENGTH];
int quantity;
printf("请输入产品名称:");
scanf("%s", name);
printf("请输入产品数量:");
scanf("%d", &quantity);
addProduct(name, quantity);
break;
case 2:
// 移除产品
printf("请输入需要移除的产品名称:");
scanf("%s", name);
printf("请输入需要移除的数量:");
scanf("%d", &quantity);
removeProduct(name, quantity);
break;
case 3:
// 显示所有产品
showProducts();
break;
case 4:
// 退出程序
printf("再见!\n");
return 0;
default:
printf("无效的选择,请重试\n");
break;
}
}
return 0;
}
```
以上代码实现了以下功能:
1. 添加产品到仓库中;
2. 移除仓库中的产品;
3. 显示仓库中的所有产品;
4. 退出程序。
你可以根据自己的需求修改这个代码,添加更多的功能。
相关推荐
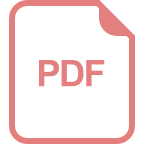














