C# 读取CSV文件并显示到DataGrid
时间: 2024-12-13 20:22:45 浏览: 27
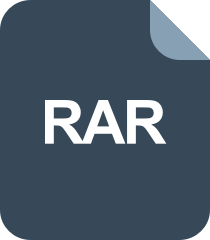
C#/WPF Csv操作:读写,增删改查

在C#中,可以使用`System.Data`命名空间下的`DataTable`和`TextFieldParser`类来读取CSV文件,并将其内容显示在`DataGridView`上。以下是一个简单的步骤概述:
1. 引入所需库:
```csharp
using System;
using System.IO;
using System.Data;
using Microsoft.VisualBasic.FileIO;
```
2. 定义CSV文件路径和DataGrid对象:
```csharp
string csvFilePath = "path_to_your_csv_file.csv";
DataGridView dataGridView = new DataGridView();
```
3. 读取CSV文件:
```csharp
// 创建一个字符串数组,用于存储每行数据
var lines = File.ReadAllLines(csvFilePath);
// 使用TextFieldParser解析CSV数据
string[] headers;
if (File.Exists(csvFilePath))
{
using (TextFieldParser parser = new TextFieldParser(csvFilePath))
{
parser.TextFieldType = FieldType.Delimited;
parser.SetDelimiters(",");
// 获取列标题
if (!parser.HasHeaderRecord)
{
throw new Exception("CSV file does not contain a header row");
}
else
{
headers = parser.ReadFields();
}
// 逐行读取并填充DataTable
DataTable dataTable = new DataTable();
foreach (string line in lines.Skip(1)) // 跳过表头行
{
string[] fields = parser.ReadFields();
DataRow newRow = dataTable.NewRow();
for (int i = 0; i < headers.Length; i++)
{
newRow[headers[i]] = fields[i];
}
dataTable.Rows.Add(newRow);
}
}
}
else
{
throw new FileNotFoundException("CSV file not found", csvFilePath);
}
// 将DataTable绑定到DataGridView
dataGridView.DataSource = dataTable;
```
4. 显示DataGrid:
```csharp
// 添加列标题到DataGrid的第一行
dataGridView.Columns.AddRange(headers.Select(h => new DataGridViewTextBoxColumn { DataPropertyName = h }));
// 设置窗口大小和可见性
dataGridView.Dock = DockStyle.Fill;
Form mainForm = new Form();
mainForm.Controls.Add(dataGridView);
mainForm.ShowDialog();
```
阅读全文
相关推荐
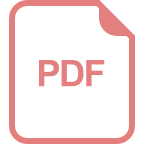
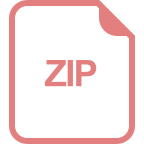
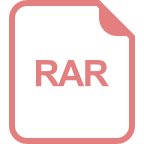
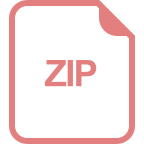
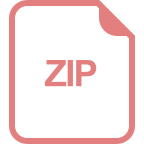
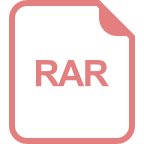
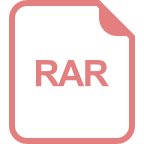
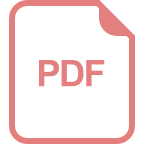
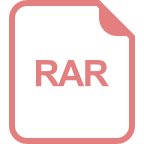
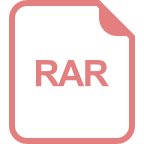
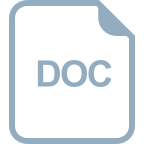
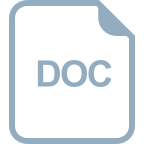
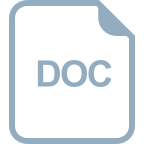
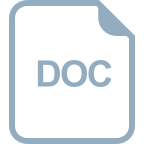
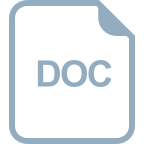
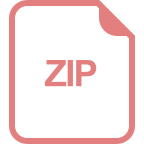
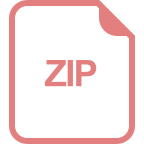
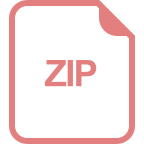