ts中interface
时间: 2024-06-14 21:05:21 浏览: 84
在TypeScript中,接口(interface)用于定义一个类的形状或对象的结构。接口可以包含属性、方法和索引签名等成员。
以下是一个使用接口定义类的形状的例子:
```typescript
interface Shape {
color: string;
}
class Circle implements Shape {
color: string;
radius: number;
constructor(color: string, radius: number) {
this.color = color;
this.radius = radius;
}
getArea(): number {
return Math.PI * this.radius * this.radius;
}
}
let circle = new Circle("red", 5);
console.log(circle.color); // 输出:red
console.log(circle.getArea()); // 输出:78.53981633974483
```
在上面的例子中,我们定义了一个接口`Shape`,它包含一个`color`属性。然后我们创建了一个`Circle`类,实现了`Shape`接口,并添加了一个`radius`属性和一个`getArea`方法。最后,我们创建了一个`Circle`类的实例,并访问了它的属性和方法。
另外,接口还可以继承其他接口,创建出多个接口的合成接口。以下是一个使用接口继承的例子:
```typescript
interface Shape {
color: string;
}
interface PenStroke {
penWidth: number;
}
interface Square extends Shape, PenStroke {
sideLength: number;
}
let square: Square = {
color: "blue",
sideLength: 10,
penWidth: 5.0
};
console.log(square.color); // 输出:blue
console.log(square.sideLength); // 输出:10
console.log(square.penWidth); // 输出:5.0
```
在上面的例子中,我们定义了三个接口:`Shape`、`PenStroke`和`Square`。`Square`接口继承了`Shape`和`PenStroke`接口的属性,然后我们创建了一个`Square`类型的对象,并给它的属性赋值。
阅读全文
相关推荐
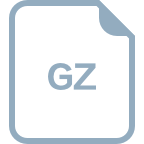
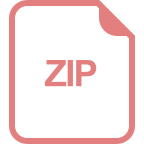
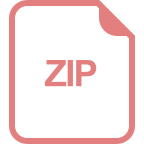














